Backend Basics For Everyone
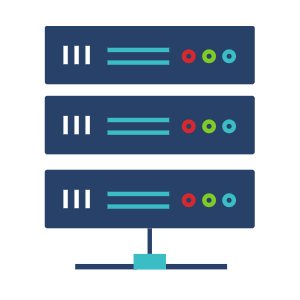
Concepts:
1. Backend Programming Introduction
2. Backend Frameworks and Libraries
25. Content Delivery Networks (CDNs)
1. Backend Programming Introduction
Backend development is the heart of any web application, and it is responsible for server-side processing of requests, data storage, and retrieval, and also for responding to client requests with the necessary data.
Backend programming is the process of writing code that runs on servers to support the functionality of web and mobile applications. It involves creating and managing databases, APIs, and server-side logic that are responsible for handling user requests and generating responses.
We will cover the following topics related to backend programming
- Backend Programming Languages
- Databases
- APIs
- Real World Examples
1. Backend Programming Languages
1. Python: It is a versatile and easy-to-learn language. Python is commonly used for building web applications, data analysis, and machine learning.
2. Java: It is a widely used language for building enterprise-level applications. Java is known for its scalability and high performance.
3. Node.js: It is a popular language that is used for building scalable and high-performance web applications. Node.js is built on top of JavaScript and is known for its speed and efficiency.
4. Ruby: It is a popular language that is used for building web applications. Ruby on Rails is a popular web framework that is built using Ruby.
Databases
A database is a structured data that is stored in an electronic device. Databases are used to store and manage data that is used by web and mobile applications.
1. MySQL: It is an open-source relational database management system. MySQL is widely used for building web applications.
2. PostgreSQL: It is a powerful and open-source object-relational database system. PostgreSQL is known for its reliability and scalability.
3. MongoDB: It is a popular NoSQL database that is used for building web applications.
APIs
An API (Application Programming Interface) is a set of rules that defines how different software components should interact with each other. APIs let the different applications to contact with each other.
Some of the most popular APIs used in backend programming are:
1. REST APIs: It is a popular API used for building web applications. REST APIs use HTTP protocol to transmit data and are known for their simplicity and scalability.
2. GraphQL:It is a query language used to interact with APIs. GraphQL is known for its flexibility and efficiency.
For example, Companies like E-commerce applications such as Amazon, eBay, and Alibaba use backend programming to manage product data, handle customer orders, and generate invoices.
In conclusion, Backend programming is an essential part of web and mobile application development. It involves creating and managing databases, APIs, and server-side logic that are responsible for handling user requests and generating responses.
2. Backend Frameworks and Libraries
Backend frameworks and libraries are tools that help developers to build web applications more efficiently, by providing pre-written code and functionality, and reducing the amount of custom code that needs to be written. In this tutorial, we will cover some popular backend frameworks and libraries, starting from the basics and progressing to more advanced topics.
1. Node.js: Used for building server-side applications using JavaScript.
2. Express.js: A minimal and flexible Node.js web application framework used for building web applications and APIs.
3. Ruby on Rails: A popular web application framework written in the Ruby programming language.
4. Django: A high-level Python web framework
5. Flask: A lightweight Python web framework
6. Laravel: Laravel
These frameworks can help developers to build web applications more efficiently by providing pre-written code and functionality, and reducing the amount of custom code that needs to be written.
3. Server
A server is a computer or computer program that provides services to other computer programs or devices on a network. It can be thought of as a "central hub" that manages and distributes resources and data.
Servers work by listening for requests from client devices, processing those requests, and sending back the appropriate response. For example, a web server will listen for requests for web pages from client devices (such as laptops, smartphones, or tablets), and then send back the requested web page.
1. Web servers: These servers host websites and web applications, and serve web pages to client devices. (Apache, Nginx, Microsoft IIS)
2. File servers: These servers store and manage files, and allow client devices to access those files over a network. (Windows File Server, FreeNAS, Nextcloud)
3. Database servers: These servers store and manage databases, allowing client devices to access and modify data stored in the database. (MySQL, PostgreSQL, Microsoft SQL Server)
4. Mail servers: These servers handle email messages, sending and receiving emails to and from client devices. (Microsoft Exchange, Postfix, Dovecot)
5. Game servers: These servers host multiplayer games, allowing players to connect and play with each other over the internet.: write examples in partanthesis. (Minecraft server, Counter-Strike server, World of Warcraft server)
4. API
An API, or Application Programming Interface, comprises a collection of protocols, routines, and tools that allow developers to create software applications.
It enables communication and data exchange between two separate software systems or applications. APIs are used to define how software components should interact and communicate with each other.
An API works by providing a set of rules for communication between different software applications. When a developer creates an API, they define how the application will be used, what data can be requested or provided, and how it should be formatted. This information is made available to other developers through the API documentation. Developers can then use the API to integrate it into their own software and make requests for data or functionality.
Types of APIs
1. RESTful APIs: These APIs are based on the REST architecture and use HTTP requests to GET, PUT, POST, and DELETE data. They are simple to use and widely adopted, making them the most popular type of API.
2. SOAP APIs:These APIs use the XML format for data exchange and rely on the SOAP protocol. They are used for complex applications and can be slower than RESTful APIs.
3. GraphQL APIs: These APIs provide a more flexible way to query data and allow developers to specify exactly what data they want. They are growing in popularity and are often used in modern web applications.
Use Cases:
Integrating with third-party services: APIs are often used to integrate with third-party services such as payment gateways, social media platforms, and weather services.
Building mobile apps: APIs are used to build mobile apps that can access data from a server. For example, a weather app can use an API to get real-time weather data.
Automating workflows: APIs can be used to automate workflows and create custom integrations between different software applications.
Developing web applications: APIs can be used to build web applications that communicate with a server in real-time. For example, a chat application can use an API to send and receive messages.
You can use several programming languages and frameworks to develop an API. Such as, Exprees.js Framework for Node.js, Ruby on Rails for Ruby, Spring for Java, etc.
5. Database
What is Database
A database is a collection of organized data that can be easily accessed, managed, and updated. It is a critical component of many software applications, including backend systems, websites, and mobile apps. A database stores data in a structured format, making it easy to search, sort, and manipulate the data.
Types of Databases
1. Relational Database: A relational database stores data in tables that are related to each other through common fields. This type of database is widely used and is the basis for SQL databases such as MySQL, PostgreSQL, and Oracle.
2. NoSQL databases: NoSQL databases are designed to handle unstructured data such as documents, graphs, and key-value pairs. They are often used for big data applications and real-time data processing.
3. Object-oriented databases: Object-oriented databases store data in the form of objects, which are instances of classes that represent real-world objects. They are often used for complex data structures and for applications that require a high degree of flexibility.
4. Graph databases: A graph database stores data in the form of nodes and edges, which represent relationships between data elements. They are often used for social networks, recommendation systems, and other applications that require complex data relationships.
6. Client
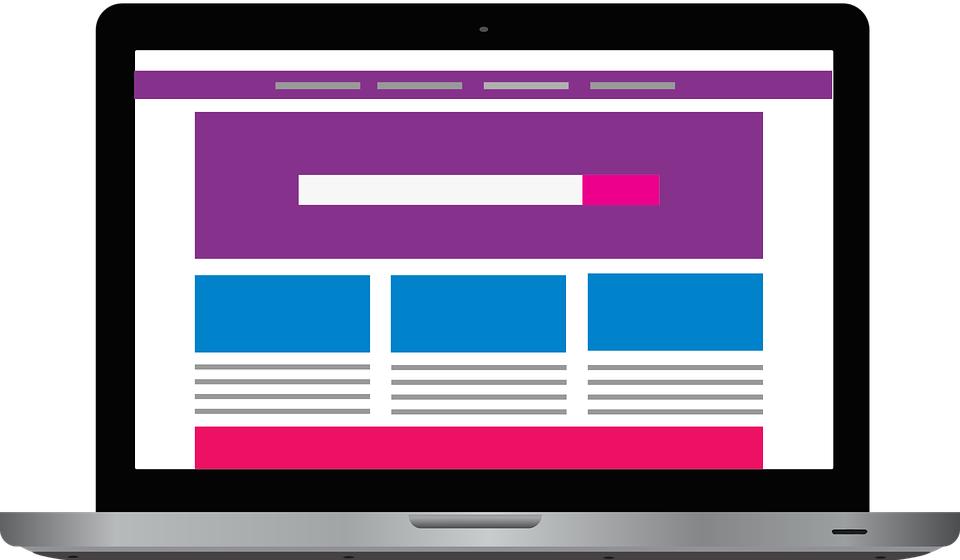
In backend terminology, a client typically refers to a device or software application that requests services or data from a server.
For example, in a client-server architecture, a client would be a device (mobile, computer, etc.) or software application that sends a request for data or services to a server. The server then processes the request and sends back the requested data or services to the client.
In web development, the client is often a web browser that sends requests to a web server for web pages or other resources. The web server then responds to the request by sending the requested resources back to the client's web browser.
7. Middleware
Middleware is a software component that sits between two applications or systems to facilitate communication and data exchange between them. It is a piece of code that intercepts requests and responses between applications and adds additional functionality to them. Middleware can be used for various purposes, such as logging, authentication, data transformation, and error handling.
Middleware works by intercepting incoming requests from clients and outgoing responses from servers. It then processes the request or response and performs any necessary actions, such as adding authentication tokens or transforming data formats. Once the processing is complete, the middleware passes the request or response back to the appropriate application or system.
The main reason to use middleware is to add additional functionality to an application or system without modifying the core code. This can help improve scalability, performance, and security, as well as making it easier to integrate with other applications or systems.
There are different ways to implement middleware depending on the programming language and framework being used. In general, middleware can be implemented using a middleware function or middleware class that takes in the request and response objects and performs the necessary processing. This middleware function or class can be added to the application's request pipeline to intercept and process incoming requests and outgoing responses. Middleware can also be implemented as a separate standalone service that communicates with the application or system via APIs or other integration methods.
8. REST and SOAP APIs
REST and SOAP are two popular architectural styles for building APIs that allow different applications or systems to communicate with each other over the internet or a network
REST (Representational State Transfer) is an architectural style that uses HTTP (Hypertext Transfer Protocol) to build web services. RESTful APIs use a set of rules (or constraints) to define how data should be transferred between client and server. It relies on stateless, client-server architecture where the server provides resources, and clients access these resources using HTTP requests. RESTful APIs use HTTP methods such as GET, POST, PUT, DELETE, etc., to manipulate resources, and the data is usually returned in JSON or XML format.
REST APIs are typically easier to implement, lightweight, and scalable. They use HTTP, which is a widely adopted protocol, and can be easily integrated with other web technologies such as HTML, CSS, and JavaScript. RESTful APIs are also more flexible in terms of data format and support a wide range of media types.
SOAP (Simple Object Access Protocol) is a protocol that uses XML to transfer data between client and server. It provides a standard way of communication between different systems and supports different programming languages and platforms. SOAP APIs use a set of rules (or specifications) to define how data should be transferred between client and server. SOAP APIs use XML to encode the data and the message is usually transmitted over HTTP, SMTP, or TCP.
SOAP APIs, on the other hand, are more secure and reliable. They provide a standardized way of communication between different systems and have built-in error handling and message validation. SOAP APIs are also more suitable for complex transactions and support more advanced features such as security, transactions, and reliability.
9. Authentication
Authentication is the process of verifying the identity of a user or application and ensuring that they have the necessary permissions to access certain resources or perform specific actions within a system. Authentication typically involves verifying a user's identity using credentials such as a username and password, a token, or a digital certificate.
To develop authentication in an application or system, there are several steps that need to be taken:
1. Define the authentication requirements: Identify the specific resources or actions that require authentication and determine the level of security needed.
2. Choose an authentication mechanism: Choose the authentication mechanism that best suits the application or system requirements, such as basic authentication, token-based authentication, OAuth 2.0, or OpenID Connect.
3. Implement the authentication mechanism: Implement the chosen authentication mechanism in the application or system, using the appropriate libraries, frameworks, or tools. This involves creating authentication endpoints, handling authentication requests, and verifying user credentials.
4. Secure the authentication process: Secure the authentication process by using best practices such as password hashing, rate limiting, and SSL/TLS encryption to prevent attacks such as brute force, man-in-the-middle, or session hijacking.
5. Test and refine the authentication mechanism: Test the authentication mechanism thoroughly, including negative and edge cases, and refine it as needed to ensure that it meets the security and usability requirements of the application or system.
There are several tools and frameworks available for developing authentication in applications or systems.
1. Auth0
2. Firebase Authentication
3. Passport.js
4. Spring Security
5. Django Authentication
10. Authorization
Authorization is the process of granting or denying access to resources or actions within a system, based on the user's identity, role, or permissions. Authorization typically comes after authentication, which verifies the user's identity and ensures that they have the necessary credentials to access the system.
Authorization works by defining rules and policies that determine which resources or actions a user or application can access. These rules are usually defined by the system administrator or the application developer and are enforced by the system's security mechanisms.
11. SSL
SSL (Secure Sockets Layer), also known as TLS (Transport Layer Security), is a protocol used for secure communication over the internet. It provides a secure channel between two parties, such as a client and a server, by encrypting the data sent between them.
SSL works by using a combination of public and private keys to encrypt and decrypt data. When a client requests a secure connection to a server, the server responds with a public key certificate that contains its public key. The client then uses the public key to encrypt data that is sent to the server, and the server uses its private key to decrypt the data.
SSL plays a critical role in backend applications because it ensures that data transmitted between the client and the server is secure and cannot be intercepted or tampered with by unauthorized parties. In a backend application, SSL is typically used to secure communication between the client and the server, such as when transmitting sensitive user data, authentication credentials, or financial information.
12. MVC
MVC (Model-View-Controller) is a design pattern used in software development that separates an application into three interconnected components: the model, the view, and the controller.
The model component of the MVC architecture is responsible for managing the application's data and implementing the business logic.
The view component represents the user interface and is responsible for displaying the data to the user.
The controller acts as a mediator between the model and the view components and is responsible for handling user input and updating the model and view accordingly.
MVC works by decoupling the application's components, which allows for greater flexibility and easier maintenance. Each component has a specific responsibility, and changes to one component can be made without affecting the others. For example, changes to the model do not require changes to the view or controller.
MVC is commonly used in backend development because it provides a clear separation of concerns between the data model, the user interface, and the application logic. This separation makes it easier to manage complex applications and to scale the application as the user base grows.
In a typical backend MVC architecture, the model represents the data and business logic of the application, such as database queries and data manipulation. The view is responsible for rendering data to the user, such as HTML templates or JSON responses. The controller handles user requests, processes data from the model, and updates the view accordingly.
13. Cache
Caching refers to storing frequently accessed data or computations in a cache, so that they can be quickly retrieved instead of being repeatedly fetched from a slower data source such as a database. Caching can significantly improve the performance of backend applications, especially for applications that involve complex and time-consuming computations or data retrieval operations.
For example, let's say that we have a backend application that serves weather information to users based on their location. The application retrieves the weather data from an external API, which can be slow and have a limited number of requests per second. To improve performance, we can implement caching so that the application stores the weather data in a cache for a certain amount of time (e.g., 5 minutes), and returns the cached data to subsequent requests that are made within that time period.
So, when a user requests weather information for a specific location, the application first checks the cache to see if the data for that location is already stored. If it is, the application retrieves the data from the cache and returns it to the user, without needing to make a new request to the external API. If the data is not in the cache, the application retrieves it from the API, stores it in the cache, and returns it to the user.
14. DevOps
DevOps is a set of practices that combines software development (Dev) and information technology operations (Ops) to optimize the entire software development lifecycle. DevOps aims to shorten the development lifecycle and provide continuous delivery with high software quality, stability, and reliability. In simpler terms, DevOps is a culture or methodology that promotes collaboration between developers and operations teams to build, test, and release software quickly and efficiently.
DevOps works by automating the software development process and providing tools for continuous integration (CI), continuous delivery (CD), and continuous deployment (CD). The key principles of DevOps include collaboration, automation, monitoring, and continuous improvement. By working together, developers and operations teams can streamline the software development process, identify and fix issues quickly, and deliver high-quality software to users faster.
15. Docker
Docker is an open-source platform that enables developers to build, package, and deploy applications in a lightweight and portable manner. It allows you to create "containers" that include all the dependencies and configurations needed to run your application, which makes it easier to deploy and run applications in different environments.
Docker works by using containerization technology to create a virtualized environment that runs applications in an isolated and portable manner. A container is an isolated environment that includes all the dependencies and configurations needed to run an application, such as the operating system, runtime, libraries, and application code. Containers are lightweight, portable, and easy to deploy, which makes them ideal for running applications in different environments, such as development, testing, and production.
16. HTTP
HTTP, or Hypertext Transfer Protocol, is a protocol used for transmitting data over the internet. It is the foundation of communication on the World Wide Web and enables web browsers to request and receive web pages and other resources from web servers.
Working of HTTP
1. An HTTP request is sent by a client, which is typically a web browser, to a server, requesting a particular resource, such as a web page or an image.
2. The server processes the request and sends an HTTP response back to the client, which includes the requested resource.
3. The client receives the HTTP response and displays the resource in the web browser.
HTTP requests and responses consist of a header and a body. The header contains metadata about the request or response, such as the HTTP method (e.g. GET, POST), the URL, and the content type. The body contains the data being transmitted, such as the HTML code of a web page or the binary data of an image.
HTTP methods, also known as HTTP verbs, are used to indicate the desired action to be performed on a resource identified by a URL.
GET: Retrieve a resource from the server.
POST: Submit data to the server.
PUT: To Update an existing data on the server.
DELETE: Delete a resource on the server.
HEAD: Retrieve metadata about a resource from the server.
OPTIONS: Retrieve information about the server's capabilities.
PATCH: Update part of an existing resource on the server.
17. Nginx, Apache
Nginx and Apache are both popular web servers that are often used in backend development to serve web content and handle requests from clients.
Apache is a mature and widely-used open-source web server that has been around since the mid-1990s. It can handle a wide variety of tasks, including serving static and dynamic content, proxying requests to other servers, and implementing custom authentication and access control.
Nginx (pronounced "engine-x") is a newer web server that was designed from the ground up to be highly scalable and efficient. It is often used as a reverse proxy or load balancer, distributing incoming requests to multiple backend servers to improve performance and reliability.
Both Apache and Nginx can be used to host websites and web applications, and they both support a wide range of programming languages and technologies. In addition, both servers can be extended with modules and plugins to add additional functionality or integrate with other tools and systems.
18. Cloud
Cloud computing involves providing access to computing resources like servers, storage, software, and analytics over the internet, commonly known as "the cloud." These resources can be utilized on-demand, allowing users to scale their usage according to their needs and only pay for the resources they consume.
Cloud computing works by providing virtual resources that can be easily allocated and de-allocated by users as needed, without worrying about hardware maintenance. Cloud providers offer various services, such as data storage, security, and monitoring to manage applications and data in the cloud efficiently.
Some examples of cloud computing providers include Amazon Web Services (AWS), Microsoft Azure, Google Cloud Platform, IBM Cloud, and Oracle Cloud. These providers offer a range of services, including infrastructure as a service (IaaS), platform as a service (PaaS), and software as a service (SaaS), among others. Many companies and organizations use cloud computing to host their applications and store their data, as it offers scalability, flexibility, and cost-effectiveness compared to traditional on-premise solutions.
19. Postman
Postman is a popular API development tool that allows developers to design, test, and document APIs quickly and easily. It can be used to send requests to a web server, receive responses, and inspect the results.
In the backend development, Postman is often used by developers to test APIs that they have developed or are using in their applications. By testing APIs using Postman, developers can ensure that their API is functioning as expected and can catch any bugs or issues before deploying it to production.
The main purpose of Postman is to simplify the process of testing and working with APIs. With Postman, developers can easily create requests and specify the necessary parameters, headers, and body data. They can then send these requests to the API and examine the response data. This helps to identify and resolve any issues with the API quickly.
Postman works by providing a user-friendly interface for creating and sending requests to an API. Developers can specify the HTTP method (e.g., GET, POST, PUT, DELETE), the URL, and any necessary parameters or headers. Postman then sends the request to the API and displays the response data, including the HTTP status code, headers, and body.
20. Firebase
Firebase is a platform developed by Google that provides a suite of tools and services for building and managing web and mobile applications. Firebase offers a range of features, including authentication, real-time database, cloud storage, cloud messaging, and more. These tools make it easier for developers to build and manage their applications, without having to worry about infrastructure or backend services.
To use Firebase in your backend application, you'll need to create a Firebase project and integrate Firebase SDKs into your application code. Firebase provides SDKs for a range of platforms, including web, iOS, Android, and more.
Once you've integrated Firebase into your application, you can start using its various features and services in your backend code. For example, you can use the Firebase Admin SDK to access Firebase services from a server, or you can use Firebase Cloud Functions to write and deploy backend logic. The specific steps for integrating Firebase into your application will depend on the platform and programming language you're using, but Firebase provides extensive documentation and tutorials to help you get started.
21. Cookies and Sessions
Cookies and sessions are both mechanisms used to store and manage user data in web applications.
Cookies are small text files that are saved on a user's device when they visit a website. They keep track of the user's activity on the site, such as login information and preferences.
Sessions are similar, but the data is stored on the server instead of the user's device. When a user logs in, the server creates a session and assigns a unique ID to it. This ID is then used to link the user's activity to the stored data.
The main difference is where the data is stored - cookies on the user's device, sessions on the server.
22. Hosting
Hosting refers to the process of storing and serving website content on a server that is accessible over the internet. In order for a website to be available to the public, it needs to be hosted on a server that can handle requests from web browsers and deliver the necessary files and data.
There are many hosting providers available that offer a range of hosting options, from basic shared hosting to dedicated servers and cloud hosting.
Bluehost, GoDaddy, SiteGround, HostGator, AWS are the popular Hosting providers.
23. DNS
DNS is a system that translates website names, like www.example.com, into a unique code that computers can understand, called an IP address, such as 192.0.2.1. When someone enters a website name in their browser, the browser asks a DNS server to find the IP address associated with that name. Once the IP address is found, the browser uses it to connect to the server hosting the website and retrieve its content.
DNS uses a system of servers to find the IP address associated with a website name. When someone types a website name into their browser, the browser first checks a local DNS server provided by their internet provider. If it's not found there, the request goes to a root DNS server, which sends it to a top-level domain server (like .com). The TLD server then sends it to the DNS server that knows about that specific website. This process continues until the IP address is found and returned to the browser.
24. Browser
A web browser is a program that lets you see websites and other online stuff. You type a website's address into the browser, and it asks the website's computer to send it the page. The browser then reads the page's code and shows you the page on your device. You can click on links, fill out forms, and see pictures, videos, and audio. Popular web browsers include Google Chrome, Mozilla Firefox, Apple Safari, and Microsoft Edge.
At a high level, a web browser is a software application that allows users to access and view content on the internet. This content can be in the form of web pages, images, videos, audio, and other types of digital media. The browser works by using the Hypertext Transfer Protocol (HTTP) to request web pages from web servers, which then send back the requested content in the form of HTML, CSS, and JavaScript code. The browser then interprets and renders this code to display the content on the user's device. Additionally, web browsers enable users to interact with web pages, navigate through different pages, and perform other functions such as searching the web and managing bookmarks.
25. Content Delivery Networks (CDNs)
A Content Delivery Network (CDN) is a group of servers located all around the world that work together to deliver website content to users quickly and reliably.
They store copies of website content on these servers in different locations, so when a user requests a file, it's delivered from the server closest to them. This reduces the distance data has to travel, making websites faster.
CDNs also use different methods to improve performance, like compressing files and using advanced routing. Some examples of popular CDNs are Akamai, Cloudflare, Amazon CloudFront, and Fastly. Many websites use CDNs to deliver content quickly and reliably to their users.
26. Git and Github
Git is a tool that helps developers keep track of changes to their code, work with others, and manage different versions of their project.
GitHub is a website where developers can store their code and collaborate with others.
They can use Git to track changes to their code and then upload those changes to GitHub, where others can see them and work together on the project. Git and GitHub are important tools for building software because they help developers work together and keep their code organized.
27. OS
An Operating System (OS) is like the boss of a computer. It makes sure the computer's hardware and software can work together, and lets people use the computer to do things like run programs and browse the internet.
The OS also decides how much of the computer's resources, like memory and storage space, each program can use. And it shows people what's happening on the computer screen and lets them control it using a mouse, keyboard or touchpad.
At a high level, an operating system (OS) is a software that manages a computer's hardware and software resources. It acts as an interface between the user and the computer, and ensures that the hardware and software components of the computer system work together effectively.
Each operating system has its own features, advantages, and limitations. The choice of operating system depends on the specific needs of the user and the type of application being used.
The popular OS are: Windows, macOS, Linux, Unix, Android, iOS