C Programming Tutorial - webdevemonk
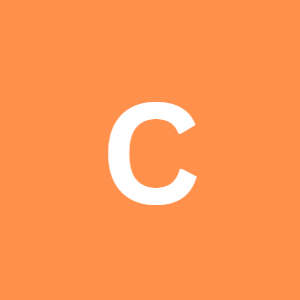
Unlock the power of programming with our comprehensive tutorial, The Building Blocks of Computer Programming Designed for beginners, it covers all the essential concepts.
Concepts:
1. C Introduction
Welcome to the world of C programming! C is a high-level programming language that is widely used in a variety of applications, including operating systems, device drivers, and scientific simulations. It is known for its efficiency and flexibility, making it a popular choice among professionals and students alike.
C is a programming language that is widely used in a variety of computer applications. Some common areas where C is used include:
1. Operating systems: C is often used to create the core of operating systems, such as Windows and Linux, due to its efficiency and ability to work closely with hardware.
2. Device drivers: C is often used to write device drivers, which are programs that allow the operating system to communicate with hardware devices such as printers and keyboards.
3. Gaming: Many video games are written in C due to its ability to handle complex graphics and real-time gameplay.
Environment Setup
To set up your environment for C programming, you will need to install a C compiler and a text editor
1. Choose a C compiler: There are several C compilers available, including GCC (GNU Compiler Collection) and Visual C++. GCC is a free, open-source compiler that is available on Linux, Mac, and Windows.
2. Install the C compiler: Follow the instructions for your chosen compiler to install it on your system.
3. Choose a text editor: A text editor is a program that allows you to write and edit text files. Some popular text editors for C programming include Notepad, Sublime Text, and Atom. Choose the text editor that you are most comfortable with.
4. Install the text editor: Follow the instructions for your chosen text editor to install it on your system.
Once you have installed a C compiler and text editor, you are ready to start writing and compiling C programs. To write a C program, open your text editor and create a new file. Type your C code into the file, save it with a ".c" extension, and then compile it using your C compiler.
2. C Syntax
The syntax of the C programming language is the set of rules that define how a C program is written and structured.
1. C is a case-sensitive language, which means that the names of variables, functions, and keywords must be written in the correct case. For example, "int" and "INT" are not the same thing in C.
2. Line termination: In C, each statement must be terminated with a semicolon (;).
3. Block structure: C uses curly braces ({ and }) to define blocks of code. This code block begins with an opening curly brace and ends with a closing curly brace.
The basic structure of a C program
1. Preprocessor directives: These are lines that begin with a pound sign (#) and are used to include header files and define macros.
2. Function prototypes: These are declarations of functions that are used in the program.
3. Global variables: These are variables that are declared outside of any function and are available to all functions in the program.
4. The 'main()' function: This is the entry point of the program and is where execution begins.
5. Local variables: These are variables that are declared inside a function and are only available within that function.
6. Statements: These are the commands that are executed by the program.
3. C Output
In C, you can use the printf() function from the stdio.h library to output text, numbers, and other data types to the console.
#include<stdio.h>
int main(){
printf("Hello World"); // Hello World
return 0;
}
The output of the above C example program is "Hello World"
4. C Comments
Comments are lines of code that are ignored by the compiler and are used to provide explanations or notes in the source code. Comments are useful for documenting your code and making it easier to understand for other developers.
In C, there are two types of comments: single-line comments and multi-line comments.
// single line comment
/* this is a
multi-line comment
*/
5. C Variables
In C, a variable is a storage location that is used to hold a value or a reference to a value. Variables are used to store data that can be manipulated and accessed by the program.
// declaring variables
int a; // integer variable that can store one single integer value
float num; // float variable that can store one single decimal value
char ch; // character variable that can store one single character value
You can assign immediately, or after declaration of the variable based on your need
int a;
a = 10;
int number = 30;
If multiple variables have same data type then you can follow this syntax
int a, b, c = 10;
You can access them with its 'name' and'format specifiers' of the specific data type
#include<stdio.h>
int main(){
int a = 10;
float num = 25.5;
char ch = 'R';
printf("%d \n", a);
printf("%f \n", num);
printf("%c \n", ch);
return 0;
}
// Output
/*
10
25.500000
R */
The '\n' character is used for a new line in C program.
In C, format specifiers are used in the 'printf()' function to specify how a value should be formatted and displayed. Each type of data has a corresponding format specifier that you can use to control how the data is displayed.
In C, there are a few rules to follow when declaring and using variables:
1. Variable names only begin with a letter or an underscore (_).
2. Variable names are case-sensitive. This means that "age" and "Age" are considered to be different variables.
3. Variable names cannot be the same as C keywords (such as "int", "char", or "while").
4. Variables must be declared before they are used. This means that you must specify the data type and name of the variable before you can assign a value to it.
6. C Data Types
In C, data types are used to specify the type and size of variables. C has a variety of built-in data types, including integers, floating-point numbers, characters, and more.
1. int:
In C this data type used to store integers (2,56,79). The size of an int variable depends on the machine, but it is typically at least 16 bits.
2. float:
In C this data type used to store single-precision floating-point numbers. A float variable is a 4-byte value that can hold decimal values with a precision of about 7 decimal digits.
3. char:
In C this data type used to store characters. A char variable is a single byte in size and can hold any character in the ASCII character set.
4. double:
In C this 'double' is a data type that represents a double-precision floating-point value. It is often used to store values with a larger range or greater precision than the 'float' data type.
void main() {
int num = 79;
float fltNum = 23.99524;
char character = 'V';
double number = 2.718281828459045;
long longNum= 93927;
printf("num = %d \n", num);
printf("fltNum = %f \n", fltNum);
printf("character = %c \n", character);
printf("number = %lf \n", number);
printf("longNum = %ld \n", longNum);
}
// Output
/*
num = 79
fltNum = 23.995239
character = V
number = 2.718282
longNum = 93927
*/
7. C Input
In C, you can use the 'scanf()' function to read input from the user. The scanf() function takes a format string and a list of pointers to variables as arguments, and it reads data from the standard input (typically the keyboard) and stores the values in the specified variables.
#include <stdio.h>
void main(){
int age;
char ch;
printf("Enter a character: \n");
scanf("%c", &ch);
printf("Enter Your age: \n");
scanf("%d", &age);
printf("Your age is: %d\n", age);
printf("The character is: %c\n",ch);
}
/*
Output:
Enter a character:
a
Enter Your age:
21
Your age is: 21
The character is: a
*/
In this example, the 'scanf()' function is used to read an integer value for the "age" variable and a character value for the "ch" variable. The values are then output to the console using the 'printf()' function.
8. C Operators
In C, operators are special symbols that perform specific operations on one, two, or three operands, and produce a result. Operators are an essential part of C programming, and they are used to perform various types of operations, such as arithmetic, logical, relational, and bitwise operations.
1. Arithmetic Operators
In C these operators perform arithmetic operations, such as addition, subtraction, multiplication, and division. Examples: '+', '-', '*', '/', '%'.
2. Logical Operators
In C these operators will perform logical operations, like as AND, OR, and NOT. Examples: '&&', '||', '!'.
3. Relational operators
In C these operators will compare two operands and return a Boolean value (true or false). Examples: ==, !=, >, <, >=, <=.
4. Assignment operators
In C these operators assign a value to a variable. Examples: '=', '+=', '-=', '*=', '/=', '%=', '&=', '|=', '^=', '<<=', '>>='.
5. Increment and decrement operators
In C these operators increment or decrement the value of a variable by 1. Examples: ++, --.
6. Conditional operator
In C these operators evaluates a condition and returns one of two values depending on the result of the condition. Example: '? :'.
7. Comma operator
In C these operators separates two or more expressions and evaluates them in left-to-right order. Example: ','.
#include <stdio.h>
int main(void) {
int a = 10;
int b = 5;
// Arithmetic operators
int c = a + b; // addition
printf("%d + %d = %d\n", a, b, c);
c = a - b; // subtraction
printf("%d - %d = %d\n", a, b, c);
c = a * b; // multiplication
printf("%d * %d = %d\n", a, b, c);
c = a / b; // division (integer division)
printf("%d / %d = %d\n", a, b, c);
// Comparison operators
if (a == b) { // equality
printf("%d is equal to %d \n", a, b);
} else {
printf("%d is not equal to %d\n", a, b);
}
if (a < b) { // less than
printf("%d is less than %d\n", a, b);
} else {
printf("%d is not less than %d \n", a, b);
}
if (a > b) { // greater than
printf("%d is greater than %d\n", a, b);
} else {
printf("%d is not greater than %d \n", a, b);
}
// Logical operators
if (a == 10 && b == 5) { // and
printf("%d is equal to 10 and %d is equal to 5 \n", a, b);
}
if (a == 10 || b == 5) { // or
printf("%d is equal to 10 or %d is equal to 5\n", a, b);
}
if (!(a == 10)) { // not
printf("%d is not equal to 10\n", a);
}
return 0;
}
// Output
/*
10 + 5 = 15
10 - 5 = 5
10 * 5 = 50
10 / 5 = 2
10 is not equal to 5
10 is not less than 5
10 is greater than 5
10 is equal to 10 and 5 is equal to 5
10 is equal to 10 or 5 is equal to 5
*/
9. C Booleans
In C, a Boolean value is a value that can be either 'true' or 'false'. C does not have a built-in Boolean data type, but you can use the '_Bool' data type or the bool data type from the 'stdbool.h' header file to store Boolean values.
Any non-zero value considered as a 'true' value and zero is considered as a 'false'
#include <stdio.h> // include the standard input/output header file
#include <stdbool.h> // include the "stdbool.h" header file
int main() {
bool flag = true; // define a Boolean variable called "flag" and assign it the value "true"
if (flag) { // if the value of "flag" is "true"
printf("True.\n");
} else { // if the value of "flag" is "false"
printf("False.\n");
}
return 0; // return 0 to indicate successful execution
}
// Output: True.
In the above C program example, the bool data type is used to declare a Boolean variable called "flag". The value of "flag" is then used in an if statement to determine which message to output to the console.
10. C if, if-else, switch
if
The 'if' statement in C allows you to specify a condition, and if that condition evaluates to 'true', a block of code will be executed.
int num = 2;
if(num > 0){
printf("I am executed"); // I am executed
}
if-else
The 'if-else' statement is similar to the 'if' statement, but it allows you to specify a block of code to be executed even if the condition is 'false'. The 'else' block will execute this false block
int num = 2;
if(num > 0){
printf("if, I am executed"); // if, I am executed
}
else{
printf("else, I am executed");
}
switch
The 'switch' statement is a control flow statement that allows you to specify multiple conditions and execute different blocks of code depending on the result of those conditions. The 'switch' statement is often used as an alternative to a series of 'if-else' statements, as it can make the code easier to read and maintain.
int num = 1;
switch (num) {
case 1:
printf("num is 1\n"); // num is 1
break;
case 2:
printf("num is 2\n");
break;
default:
printf("num is something else\n");
break;
}
In the C example program above, the 'switch' statement will evaluate the value of num and execute the corresponding block of code. The 'break' statement is used to exit the switch statement and prevent the code from falling through to the next case. The 'default' case is optional and is executed if none of the other cases match the value of num.
11. C while, do-while, for
while loop in C
A while loop allows you to repeat a block of code as long as a specific condition is true. It means the body of the while loop will always execute while the condition is always true.
while (condition) {
// code block to be executed
}
#include <stdio.h>
int main() {
int i = 1;
while (i <= 10) {
printf("Hello %d\n", i);
i++;
}
return 0;
}
/* Output:
Hello 1
Hello 2
Hello 3
Hello 4
Hello 5
Hello 6
Hello 7
Hello 8
Hello 9
Hello 10
*/
The above C code will print the numbers from 1 to 10 and print "Hello" in 10 times
do-while
A do-while loop is similar to a while loop, but the code block is guaranteed to be executed at least once, because the condition is checked after the code block has been executed.
do {
// code block to be executed
} while (condition);
#include <stdio.h>
int main() {
int num;
do {
printf("Enter a positive number: ");
scanf("%d", &num);
} while (num <= 0);
printf("Thank you!\n");
return 0;
}
/* Output:
Enter a positive number: -7
Enter a positive number: -8
Enter a positive number: 79
Thank you!
*/
The above C example code will ask the user to enter a number, and it will continue to do so until the user enters a positive number
#include <stdio.h>
int main() {
int num = 1;
do {
printf("Hello"); // Hello
} while (num <= 0);
return 0;
}
In the above example, the condition is false but it still execute one time. Becuase it execute first then it will go to check the condition.
for loop
A for loop is a concise way to write a loop that iterates over a range of values.
for (initialization; condition; increment) {
// code block to be executed
}
The initialization statement is executed before the loop starts. The condition is checked before each iteration of the loop. If the condition is true, the code block is executed, and then the increment statement is executed. This method is executed repeatedly until the condition becomes false.
#include <stdio.h>
int main() {
for (int i = 1; i <= 10; i++) {
printf("%d\n", i);
}
return 0;
}
/*
Output:
1
2
3
4
5
6
7
8
9
10
*/
The above C example program will print the numbers from 1 to 10
12. C break and continue
The 'break' statement is used to exit a loop before it complete. When a 'break' statement is encountered within a loop, the loop is immediately terminated and control is transferred to the next statement after the loop.
#include <stdio.h>
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break;
}
printf("%d\n", i);
}
printf("Done!\n");
return 0;
}
/*
Output:
1
2
3
4
Done!
*/
The above C example program will print the numbers from 1 to 10, but it will exit the loop as soon as the number 5 is encountered:
continue
The 'continue' statement is used to skip the rest of the current iteration of a loop and move on to the next iteration. When a 'continue' statement is encountered within a loop, the rest of the code in the current iteration is skipped, and control is transferred to the next iteration of the loop.
#include <stdio.h>
int main() {
for (int i = 1; i <= 10; i++) {
if (i == 5) {
continue;
}
printf("%d\n", i);
}
printf("Done!\n");
return 0;
}
/*
Output:
1
2
3
4
6
7
8
9
10
Done!
*/
The above example program code will print the numbers from 1 to 10, but it will skip the number 5
It is important to note that the 'break' and 'continue' statements can only be used within a loop. Attempting to use them outside of a loop will result in a compile-time error.
13. C Arrays
In C, an array is a collection of elements of the same data type. Arrays are very useful for storing and managing large amounts of same data.
To declare an array in C, you need to specify the type of elements it will contain and the size of the array.
data_type array_name[size];
int numbers[10];
You can also initialize an array when you declare it by specifying the values of its elements in curly braces
int numbers[5] = {1, 4, 7, 8, 9};
To access an element of an array, you use the index of the element in square brackets. Array indices start at 0, so the first element of the array has an index of 0, the second element has an index of 1, and so on.
#include <stdio.h>
int main() {
int numbers[5] = {1, 4, 7, 8, 9};
printf("The third element of the array is %d\n", numbers[2]); // The third element of the array is 7
return 0;
}
The above C code accesses the third element of the array and prints it to the screen
You can also use a loop to iterate over the elements of an array and perform an operation on each element.
#include <stdio.h>
int main() {
int numbers[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
printf("%d\n", numbers[i]);
}
return 0;
}
/*
Output:
1
2
3
4
5
*/
The above C program will print all the elements of the array
You can take elements for an array from the user using the 'scanf()' function in C, you can use a loop to iterate over the elements of the array and use the 'scanf()' function to read each element from the user.
#include <stdio.h>
int main() {
int size;
printf("Enter the size of the array: ");
scanf("%d", &size);
int numbers[size];
for (int i = 0; i < size; i++) {
printf("Enter element %d: ", i+1);
scanf("%d", &numbers[i]);
}
printf("Elements of the array: ");
for (int i = 0; i < size; i++) {
printf("%d ", numbers[i]);
}
printf("\n");
return 0;
}
// Output:
/*
Enter the size of the array: 3
Enter element 1: 5
Enter element 2: 6
Enter element 3: 8
Elements of the array: 5 6 8
*/
You can also change the element in the array using it's index position
#include<stdio.h>
int main() {
int arr[5] = {1,2,3};
printf("Before changing: ");
for(int i = 0; i < 3; i++){
printf("%d ",arr[i]);
}
arr[2] = 9;
printf("After changing: ");
for(int i = 0; i < 3; i++){
printf("%d ",arr[i]);
}
return 0;
}
// Output:
/*
Before changing: 1 2 3
After changing: 1 2 9
*/
14. C Strings
In C, a string is a sequence of characters stored in an array. Strings are useful for storing and manipulating text data.
In general we don't have a seperate data type for Strings so we use array characters.
char string_name[size];
char name[20];
The above C program, declares a string with a size of 20 characters
You can also initialize a string when you declare it by enclosing the characters in double quotes
char name[] = "John Smith";
To access a character in a string, you use the index of the character in square brackets. String indices start at 0, so the first character of the string has an index of 0, the second character has an index of 1, and so on.
#include <stdio.h>
int main() {
char name[] = "John Smith";
printf("The third character of the string is %c\n", name[2]);
return 0;
}
It is important to note that strings in C are null-terminated, which means that they are terminated by a special character called the null character ('\0'). This character is used to mark the end of the string, and it is not counted as part of the string. When you iterate over the characters of a string, you should stop when you encounter the null character.
String functions
C also provides a set of string functions in the 'string.h' header file that can be used to manipulate strings.
1. 'strlen()': returns the length of a string.
2. 'strcpy()': copies one string to another.
3. 'strcat()': concatenates two strings.
4. 'strcmp()': compares two strings.
#include <stdio.h>
#include <string.h>
int main() {
char s1[20] = "Hello";
char s2[20] = "World";
// concatenate s1 and s2 and store the result in s1
strcat(s1, s2);
// print the length of s1
printf("Length of s1: %d\n", strlen(s1));
// compare s1 and s2
if (strcmp(s1, s2) == 0) {
printf("s1 and s2 are equal\n");
} else {
printf("s1 and s2 are not equal\n");
}
return 0;
}
/*
Output:
Length of s1: 10
s1 and s2 are not equal
*/
15. C Functions
In C, a function is a block of re-usable code that performs a specific task when it is called. Functions are useful for organizing and reusing code, and they help to make your code more modular and easier to maintain.
return_type function_name(parameter_list if any) {
// function body
}
1. 'return_type': specifies the data type of the value returned by the function. If the function does not return a value, you can use the 'void' keyword.
2. 'function_name': specifies the name of the function. Function names follow the same rules as variable names in C.
3. 'parameter_list': specifies a list of parameters that the function accepts. Each parameter consists of a data type and a name. If the function does not accept any parameters, you can omit the parameter list.
#include<stdio.h>
int main() {
say_hi();
say_hi();
say_hi();
return 0;
}
void say_hi(){
printf("Hi\n");
}
/*
Output:
Hi
Hi
Hi
*/
In the above example program, the text "Hi" will print 3 times. because we call 'Say_hi' function 3 times in the 'main()' function
#include<stdio.h>
int main() {
printf("The sum of 9 and 4 is %d\n", sum(9,4));
printf("The sum of 5 and 3 is %d\n", sum(5,3));
printf("The sum of 10 and 8 is %d\n", sum(10,8));
return 0;
}
int sum(int a, int b){
int sum = a + b;
return sum;
}
// Output
/*
The sum of 9 and 4 is 13
The sum of 5 and 3 is 8
The sum of 10 and 8 is 18
*/
Whenver the 'return' statement is encounter in the function it stop executing that function and will return the value where it is called.
16. C Pointers
In C, a pointer is a specail kind of variable that stores the computer memory address of another variable. Pointers are useful for manipulating and accessing data stored in memory, and they are an essential concept in C programming.
To declare a pointer in C, you use the * operator.
type *ptr_name;
The following code declares a pointer to an integer
int *ptr;
To access the value stored in the memory location pointed by a pointer, you use the * operator. This is known as dereferencing the pointer.
For example, the following code declares an integer variable 'a' and a pointer ptr that points to 'a', and it sets the value of 'a' to 10 using the pointer.
#include <stdio.h>
int main() {
int a = 10;
int *ptr = &a; // assign the address of a to ptr
*ptr = 20; // dereference ptr and set the value of a to 20
printf("a = %d\n", a); // a = 20
return 0;
}
This code will print 'a = 20' to the screen.
You can also use pointers to pass variables by reference to a function. When you pass a variable by reference, the function can modify the original variable. To pass a variable by reference, you need to pass the address of the variable using the & operator.
#include <stdio.h>
// function prototype
void swap(int *a, int *b);
int main() {
int var1 = 10;
int var2 = 20;
printf("Before swapping: var1 = %d, var2 = %d\n", var1, var2);
swap(&var1, &var2);
printf("After swapping: var1 = %d, var2 = %d\n", var1, var2);
return 0;
}
// function
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
// Output:
/*
Before swapping: var1 = 10, var2 = 20
After swapping: var1 = 20, var2 = 10
*/
The above C code defines a function called 'swap()' that takes two pointers to integers as parameters and swaps the values pointed by the pointers. The swap() function is called in the 'main()' function, and the values of x and y are swapped.
It is important to note that pointers can be a powerful and useful tool, but they can also be complex if used improperly. In particular, you need to be careful with memory management when using pointers, and you should always make sure to deallocate any memory that you have allocated dynamically.
17. C Recursion
In C, recursion is a technique for defining a function that calls itself repeatedly until a certain condition is met. Recursion is useful for solving problems that can be divided into smaller subproblems, and it is a key concept in computer science.
To define a recursive function in C, you need to specify a base case that stops the recursion, and you need to specify a recursive case that calls the function itself with a modified set of arguments.
An example of a recursive function that finds the factorial of a specific number
#include <stdio.h>
int factorial(int n) {
if (n == 0) {
// base case: 0! = 1
return 1;
} else {
// recursive case: n! = n * (n-1)!
return n * factorial(n-1);
}
}
int main() {
int num;
printf("Enter a number: ");
scanf("%d", &num);
printf("The factorial of %d is %d\n", num, factorial(num));
return 0;
}
// Output:
/*
Enter a number: 3
The factorial of 3 is 6
*/
When you call a recursive function, the function calls itself with a modified set of arguments, and the function calls are stored on the call stack. When the base case is reached, the function calls are popped from the stack and the results are combined to produce the final result.
18. C Structures
In C, a structure is a user-defined data type that consists of a collection of related variables. Structures are useful for organizing and manipulating data that has multiple attributes, and they are an essential concept in C programming.
To define a structure in C, you use the 'struct' keyword followed by the name of the structure and the list of variables in curly braces.
struct struct_name {
type var1;
type var2;
...
};
For example, the following code defines a structure called student that represents a student with three attributes: name, age, and grade:
struct student {
char name[50];
int age;
float grade;
};
To create a variable of a structure type, you need to use the struct keyword followed by the name of the structure and the name of the variable.
struct struct_name var_name;
For example, the following code creates a structure variable called s1 of type student
#include <stdio.h>
int main() {
struct student s1 = {"John Smith", 20, 90.5};
printf("Name: %s\n", s1.name);
printf("Age: %d\n", s1.age);
return 0;
}
/*
Output:
Name: John Smith
Age: 20
*/
#include <stdio.h>
#include <string.h>
struct student {
char name[50];
int age;
float grade;
};
// function prototype
void print_student(struct student s);
int main() {
struct student s1;
strcpy(s1.name, "John Smith");
s1.age = 20;
s1.grade = 90.5;
// print the student data
print_student(s1);
return 0;
}
// function definition
void print_student(struct student s) {
printf("Name: %s\n", s.name);
printf("Age: %d\n", s.age);
printf("Grade: %.1f\n", s.grade);
}
The above code defines a structure called 'student' that represents a 'student' with three attributes: name, age, and grade. It also defines a function called 'print_student()' that takes a structure of type student as a parameter and prints the data of the student to the screen.
In the 'main()' function, the code creates a structure variable s1 of type student and initializes its variables with the data of a student. It then calls the 'print_student()' function to print the data of the student to the screen.
Name: John Smith
Age: 20
Grade: 90.5
19. C Unions
In C, a union is a user-defined data type that consists of a collection of variables that share the same memory location. Unions are useful for saving memory when you need to store different data types in the same location, and they are an essential concept in C programming.
To define a union in C, you use the union keyword followed by the name of the 'union' and the list of variables in curly braces.
union union_name {
type var1;
type var2;
...
};
#include <stdio.h>
union value {
int i;
float f;
};
int main() {
union value v;
// store an integer in the union
v.i = 10;
printf("v.i = %d\n", v.i);
// store a float in the union
v.f = 3.14;
printf("v.f = %f\n", v.f);
// the value of v.i is now invalid, because it was overwritten by v.f
printf("v.i = %d\n", v.i);
return 0;
}
// Output
/*
v.i = 10
v.f = 3.14
v.i = 0
*/
Difference between Structure and Union in C?
The main difference between a structure and a union in C is that a structure has separate memory locations for each of its variables, while a union has a single memory location shared by all of its variables.
This means that when you define a structure, the memory required to store the structure is equal to the sum of the sizes of its variables. For example, if you define a structure with two integers and a float, the memory required to store the structure will be 8 bytes (assuming that each integer takes up 4 bytes and the float takes up 4 bytes).
On the other hand, when you define a union, the memory required to store the union is equal to the size of the largest variable in the union. For example, if you define a union with an integer and a float, the memory required to store the union will be 4 bytes (assuming that the integer takes up 4 bytes and the float takes up 4 bytes).
Here is an example of a program that illustrates the difference between structures and unions in C
#include <stdio.h>
struct point {
int x;
int y;
};
union value {
int i;
float f;
};
int main() {
struct point p = {1, 2};
union value v;
printf("Size of struct point: %ld bytes\n", sizeof(struct point));
printf("Size of union value: %ld bytes\n", sizeof(union value));
// store an integer in the union
v.i = 10;
printf("v.i = %d\n", v.i);
// store a float in the union
v.f = 3.14;
printf("v.f = %f\n", v.f);
// the value of v.i is now invalid, because it was overwritten by v.f
printf("v.i = %d\n", v.i);
return 0;
}
/*
Output:
Size of struct point: 8 bytes
Size of union value: 4 bytes
v.i = 10
v.f = 3.140000
v.i = 1078523331
*/
20. C Files
In C, a file is a collection of data stored on a storage device, such as a hard drive or a USB drive. Files are used to store and manipulate data in a persistent manner, which means that the data remains in the file even after the program that created the file has finished executing.
There are two kinds of files in C language: text files and binary files. Text files contain data in the form of plain text, and they are used to store data that can be easily read and edited by humans. Binary files contain data in a binary format, and they are used to store data that is not intended to be read or edited by humans.
To work with files in C, you need to use the 'stdio.h' library, which provides a set of functions for reading and writing files. Some of the most common file manipulation functions in C are
1. 'fopen()': opens a file for reading or writing.
2. 'fclose()': closes a file that was previously opened with fopen().
3. 'fgetc()': reads a single character from a file.
4. 'fputc()': writes a single character to a file.
5. 'fgets()': reads a line of text from a file.
6. 'fputs()': writes a line of text to a file.
7. 'fread()': reads a block of data from a file.
8. 'fwrite()': writes a block of data to a file.
#include <stdio.h>
int main() {
// open a file for writing
FILE *file = fopen("data.txt", "w");
if (file == NULL) {
printf("Error opening file!\n");
return 1;
}
// write a line of text to the file
fputs("Hello, world!\n", file);
// close the file
fclose(file);
// open the file for reading
file = fopen("data.txt", "r");
if (file == NULL) {
printf("Error opening file!\n");
return 1;
}
// read a line of text from the file
char buffer[100];
fgets(buffer, 100, file);
printf("%s", buffer);
// close the file
fclose(file);
return 0;
}
// Output:
// Hello, world!
The above code opens a file called 'data.txt' for writing, writes a line of text to the file, closes the file, and then reopens it for reading. It reads a line of text from the file and prints it to the screen.
It is important to note that you need to use the 'fopen()' function with caution, because it can fail if the file does not exist or if you do not have permission to access the file. You should always check the return value of 'fopen() 'to ensure that the file was opened successfully before attempting to read or write data to the file.
21. C enum
In C, an enumeration (enum) is a user-defined data type that consists of a set of named constants. Enumerations are useful for defining a set of related constants and giving them meaningful names, and they are an essential concept in C programming.
To define an enumeration in C, you use the 'enum' keyword followed by the name of the enumeration and the list of constants in curly braces.
enum enum_name {
const1,
const2,
...
};
For example, the following code defines an enumeration called colors that represents a set of colors:
enum colors {
RED,
GREEN,
BLUE
};
To create a variable of an enumeration type, you need to use the 'enum' keyword followed by the name of the enumeration and the name of the variable.
enum enum_name var_name;
#include <stdio.h>
enum colors {
RED,
GREEN,
BLUE
};
int main() {
enum colors c = RED;
printf("Color: %d\n", c);
return 0;
}
// Output
// Color: 0
This code defines an enumeration called colors and creates an enumeration variable c of type colors. It assigns the value RED to c and prints it to the screen.
It is important to note that the constants in an enumeration are assigned consecutive integer values starting from 0 by default. However, you can also specify explicit values for the constants in the enumeration if you want.
enum fruits {
APPLE = 1,
BANANA = 2,
ORANGE = 4
};
Why to use 'enum'?
Type safety: Enumerations are a separate data type in C, which means that you can't accidentally use an enumeration value where an integer value is expected. This helps to prevent errors in your code and makes it easier to debug.
Improved readability: Enumerations allow you to give meaningful names to a set of related constants, which makes your code easier to read and understand. For example, using an enumeration to represent the months of the year is more readable than using integer constants to represent the same values.
Overall, enumerations are a useful tool for improving the readability, type safety, memory usage, and performance of your C programs.
Thank You
Thank you so much for taking the time to visit 'webdevemonk' and learn about C programming through our tutorial. We hope that you found the information helpful and that you feel more confident in your coding skills.
We strive to provide high-quality, easy-to-follow tutorials for those who are interested in learning web development and programming. We appreciate your support and hope that you continue to visit and learn with us.
Again, thank you for choosing 'webdevemonk'. We hope to see you again soon!