Computer Programming Basics
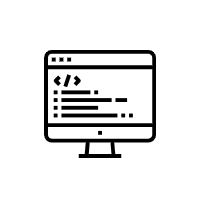
Hello folks! Welcome to our tutorial on programming language concepts. In this tutorial, we will introduce you to the fundamental concepts used in programming languages and provide real-world examples to help you understand how they are applied in practice. Whether you are new to programming or an experienced developer, this tutorial is for you. Let's get started!
Concepts:
1. Introduction to Computer Programming
32. Difference between Cloud and Data Base
1. Introduction to Computer Programming
Computer programming is the process of designing and building instructions that a computer can follow to complete a task. It is a way of telling a computer what you want it to do using a specific set of instructions.
example
Creating apps: We know, many people use apps on their phones or tablets to do things like play games, check the weather, or order food. These apps are created using computer programming languages.
Designing websites: If you visit a website, you are using a program that was created using computer programming. Websites are made up of many different elements, like text, images, and buttons, and a programmer puts all of these elements together to create the website.
Controlling robots: Some factories use robots to build products or move materials around. These robots are controlled using computer programming, which tells them what tasks to complete and how to do them.
In simple words, computer programming is used to create all kinds of programs and systems that make our lives easier and more convenient. It is an important skill to learn if you are interested in working with computers or technology.
2. Programming languages
Programming languages are used to write instructions that a computer can understand and follow. Just like we use different languages like English, Spanish, or French to communicate with people, we use different programming languages to communicate with computers.
There are many different programming languages, and each one is used for a specific purpose. For example, some programming languages are used to create websites, while others are used to create apps or games.
example
HTML: This programming language (actually it is a markup language) is used to create the structure and layout of a website. It tells the website what elements to display and where to display them.
Java: This popular programming language is often used to create apps for Android phones and tablets. It is also used to create games and other types of software.
Python: This programming language is used for a variety of purposes, including creating web servers, analyzing data, and building artificial intelligence systems.
3. Source Code
The Source code is a set of instructions that a programmer writes using a programming language. It is the "raw" code that is written and edited by a programmer, and it is usually stored in a file with a specific file extension that indicates the programming language it is written in.
Source code is used to create all kinds of programs and systems that run on computers. When a programmer writes the source code for a program, they are essentially writing a set of instructions that tell the computer what to do.
For example, if you wanted to make a simple game where you have to catch falling objects, you might write a set of instructions like this
1. Display a picture of a person on the screen
2. Display a picture of a falling object above the person
3. If the person moves their hand to the same spot on the screen as the falling object, make a "catching" sound and display a message that says "You caught the object!"
4. If the person doesn't catch the object, make a "miss" sound and display a message that says "You missed the object!"
These instructions are like the source code for your game. They tell the computer what to do and how to behave.
4. Compiler and Interpreter
These are just work like a translator between the humans and the computers
A compiler is a program that takes source code written in a programming language and converts it into a form that a computer can understand and execute. When you write source code, you are writing it in a "high-level" programming language that is easier for humans to read and understand. However, computers can only execute instructions that are written in a "low-level" language called machine code.
A compiler takes the source code and converts it into machine code that the computer can execute. This process is called "compiling."
An interpreter is a program that executes instructions written in a programming language directly, without first compiling the instructions into machine code. Interpreters read the source code and execute it line by line, translating the instructions into actions that the computer can perform.
example
When you download an app to your phone or tablet, it was probably created using a programming language like Java or C++. These languages are usually compiled into machine code before they can be run on a device.
Some programming languages, like Python and Ruby, are typically interpreted rather than compiled. This means that you can run the source code directly without first compiling it.
When you install a piece of software on your computer, it was probably compiled into machine code before it was distributed. This makes it easier for the computer to execute the instructions in the software.
5. ASCII
ASCII (American Standard Code for Information Interchange) is a standard code that represents characters (letters, numbers, symbols) as numbers. Each character is represented by a unique number, which can be stored in a computer as a series of bits (ones and zeros).
ASCII is used to represent characters in computers and other devices that use text. It is a widely-used standard, and it is supported by almost all computer systems and devices.
example
Text messaging: When you send a text message on your phone, the message is converted into ASCII code before it is transmitted to the recipient. The recipient's phone receives the message and converts the ASCII code back into the original text message.
Webpages: Assume that you visit a webpage, the text on the page is stored in ASCII code on the web server. When you request the webpage, your web browser downloads the ASCII code and displays the text on your screen.
Printing documents: Whenever you print a document, your computer sends the ASCII code for each character in the document to the printer. The printer receives the ASCII code and prints the characters on the page.
In simple terms, ASCII is an important standard that is used to represent characters in computers and other devices. It allows us to store and transmit text in a way that is understood by computers and other devices.
6. Memory Address
A memory address is a unique identifier for a specific location in a computer's memory. Memory is a type of storage that a computer uses to hold data and instructions. It is divided into many small slots, and each slot has its own unique memory address.
When a program is running on a computer, it uses memory to store and access data. The program can access a specific location in memory by using its memory address. This is similar to how you might use an address to find a specific house on a street.
Here are three examples of how memory addresses are used in the real world
Storing data: When you save a file on your computer, the computer stores the data in a specific location in memory. The memory address of that location is used to access the data when you open the file.
Running programs: When you run a program on your computer, the program is loaded into memory and its instructions are stored at specific memory addresses. The computer accesses these addresses to execute the instructions in the program.
Debugging software: When a programmer is trying to fix a problem in a program, they might use memory addresses to identify where the problem is occurring. By looking at the data stored at specific memory addresses, they can often figure out what is causing the problem.
Overall, memory addresses are an important part of how computers store and access data. They are used to identify specific locations in memory and access the data stored there.
7. Comments
In general, the comments are lines of text that are added to the source code of a program but are not executed as part of the program. Comments are used to add explanations or notes to the code, and they are usually ignored by the compiler or interpreter when the program is run.
Comments are an important part of programming because they allow programmers to add explanations and documentation to their code. This makes it easier for other people (or even the programmer themselves) to understand what the code is doing.
8. Output
Output in computer programming refers to the data that a computer program produces. For example, if you are using a computer program to do math, the output might be the answer to a math problem. Or, if you are using a computer program to play a game, the output might be the images and sounds that you see and hear on the screen.
example
1. When you use a calculator app on your phone, the numbers you enter and the result of the math calculation are all examples of output.
1. In a video game, the characters and objects on the screen, as well as the music and sound effects, are all examples of output.
1. When you search for something on the internet using a search engine, the list of websites and other information that appears on the screen is an example of output.
9. Input
Input is refers to the data that is entered into a computer program. This can include things like numbers, words, or other types of information.
example
1. When you type a message on your phone or computer, the letters and words you type are examples of input.
2. When you use a computer program to create a document or a picture, the text and images you add to the document or picture are examples of input.
3. When you use a computer program to make a purchase online, the credit card number and other personal information you enter are examples of input.
10. Variables
Variables in computer programming are like containers that store values or information. They can be used to store different types of data, such as numbers, words, or true/false statements.
These variables are mutable and temporary stored in computer memory.
1. A weather app might use a variable to store the current temperature. The variable could be called "currentTemperature" and it might store a number like 72 degrees.
2. Any game app might use a variable to keep track of the player's score. The variable could be called "playerScore" and it might store a number that increases every time the player wins a level.
3. An e-commerce website might use a variable to store the total cost of a customer's order. The variable could be called "orderTotal" and it might store a number like 45.99.
11. Data Types
In programming, data types are like categories that determine what kind of information a variable can store. Different data types allow for different kinds of values to be stored.
1. A "string" data type is used to store words or phrases. For example, in a social media app, a string variable might be used to store a user's name or bio.
2. A "number" data type is used to store numerical values. For example, in a calculator app, a number variable might be used to store the result of a math calculation.
3. A "boolean" data type is used to store true/false values. For example, in a to-do list app, a boolean variable might be used to store whether or not a task has been completed.
12. Operators
The operators are symbols that are used to perform operations on variables. These operations can include things like mathematical calculations, assignment of values, or comparison of values.
1. The "+" operator is used to add two numbers together. For example, in a shopping app, the "+" operator might be used to calculate the total cost of multiple items in a customer's cart.
2. The "-" is used to subtract. For example, in a game app, the "-" operator might be used to decrease a player's health points when they get hit by an enemy.
3. The "==" is used to check if two values are equal. For example, in a quiz app, the "==" operator might be used to check if the user's answer is correct.
13. Strings
A string is a data type that is used to store a sequence of characters, such as letters, numbers, or symbols. Strings are often used to store words or phrases ('s',T', '9', '@', etc).
example
1. In a messaging app, a string might be used to store the text of a message that a user types in.
2. A website that displays movie information, a string might be used to store the title of a movie.
3. In a virtual pet app, a string might be used to store the name that a user gives to their pet.
14. Booleans
A boolean is a data type that can have one of two values: true or false. Booleans are often used to store the result of a true/false question or to represent on/off states.
1. In a music streaming app, a boolean might be used to store whether or not the user has a premium subscription. If the user has a premium subscription, the boolean would be set to true. If not then it could be false
2. In a navigation app, a boolean might be used to store whether or not the user has enabled location services. If location services are enabled, the boolean would be set to true.If not then it could be false.
3. In a quiz app, a boolean might be used to store the result of a true/false question. If the user's answer is correct, the boolean would be set to true. If not then it could be false
15. Conditional Statements
A conditional statements are used to execute different pieces of code based on whether a certain condition is true or false. They allow the program to make decisions and take different actions based on the data it is processing.
example
1. In a weather app, a conditional statement might be used to check the current temperature. If the temperature is above 80 degrees, the app could display a message saying "It's warm outside!" or if the temperature is below 80 degrees, the app could display a message might be "It's cool outside!"
2. In a game app, a conditional statement might be used to check the player's score. If the player's score is above a certain threshold, the app could unlock a new level for the player to play. If the player's score is below the threshold, the app could display a message saying "Keep playing to unlock the next level!"
3. In a social media app, a conditional statement might be used to check the user's age. If the user is under 13 years old, the app could display a message saying "Sorry, you must be 13 or older to use this app." If the user is 13 or older, the app could allow the user to create an account.
16. Loops
Loops are a way for a computer to repeat a task multiple times. This can be really useful when we want to do the same thing over and over again, without having to type out the same code multiple times.
example
1. Let's say you have a program that displays the numbers from 1 to 10 on the screen. You could use a loop to tell the computer to print each number, one at a time.
2. A program that checks the password a user enters to make sure it is correct. If the password is incorrect, the program could use a loop to ask the user to try again until they enter the correct password.
3. A program that searches through a large database to find a particular piece of information. The program could use a loop to go through each record in the database, one at a time, until it finds the information it is looking for.
17. Functions
A function is a block of code that performs a specific task. Functions are really useful because they allow us to reuse code in different parts of our program, without having to type out the same code multiple times.
example
1. Let's say we have a program that displays a greeting to the user. We could create a function called "displayGreeting" that contains the code to display the greeting. Then, whenever we want to show the greeting to the user, we can just call the "displayGreeting" function. This is like using a recipe from a cookbook we can use the same recipe multiple times to make the same dish, without having to write out the instructions each time.
2. Assume that a program that needs to send an email. You could create a function that takes the email address, subject, and message as inputs, and then uses those inputs to send the email. This way, you only need to write the code to send an email once, and you can use the function whenever you need to send an email.
18. Classes and Objects
A class is a template or blueprint for creating objects. Generally, an object is an instance of a class.
Imagine you want to create a program that helps you keep track of your favorite movies. You could create a class called "Movie" that has information about each movie, such as its title, director, and release year. This class would be like a blueprint for creating movie objects.
Then, you could create individual movie objects using the "Movie" class as a template. For example, you could create a movie object for the film "Star Wars" using the "Movie" class. This object would have its own specific title, director, and release year. You could create additional movie objects for other films using the same "Movie" class.
example
1. You could create a class called "Person" that has information about people, such as their name, age, and address. Then, you could create individual person objects for each person you want to store information about.
2. A class called "Book" that has information about books, such as their title, author, and number of pages. Then, you could create individual book objects for each book you want to store information about.
19. Arrays
An array is a way to store a list of values in a computer program. Each value in the list is called an element, and the elements can be of different types, such as numbers, strings, or objects.
Imagine you want to create a program that keeps track of your favorite colors. You could create an array called "favoriteColors" that stores the colors you like. The array might look something like this: favoriteColors = ["red", "green", "blue"]
example
You could create an array called "studentGrades" that stores a list of grades for each student in a class. Each element in the array could be a number representing a student's grade.
An array called "shoppingList" that stores a list of items you need to buy at the store. Each element in the array could be a string representing an item on the list.
An array called "employeeRecords" that stores a list of employee objects, where each object has information about a particular employee, such as their name and job title.
20. Data Structures
A data structure is a way of organizing and storing data in a computer so that it can be accessed and modified efficiently. There are many different types of data structures, and each one is designed to support specific operations and scenarios.
example
1. An array is a data structure that stores a list of values. Each value in the list is called an element, and the elements can be of different types, such as numbers, strings, or objects. Arrays are useful for storing lists of data that need to be accessed in order, such as a list of high scores in a game.
2. A linked list is a data structure that stores a list of elements, with each element pointing to the next element in the list. Linked lists are useful for inserting and deleting elements from the list quickly, because you only need to update the pointers of the elements that come before and after the element you are inserting or deleting.
3. Linked list might be used to store a list of the user's posts on their timeline. Each element in the list could be an object representing a post, with information about the post's content, time stamp, and other details. The linked list would allow the app to efficiently insert and delete posts as needed.
4. A stack is a data structure that stores a list of elements in a last-in, first-out (LIFO) order. Stacks are useful for keeping track of a list of items that need to be processed in a specific order, such as a list of undo/redo actions in a word processor.
5. A stack might be used to implement the "undo" functionality in a text editor within the app. When the user makes a change to their text, the app could push a copy of the previous version onto the stack. If the user wants to undo their change, the app could pop the previous version off the stack and restore it.
6. A queue is a data structure that stores a list of elements in a first-in, first-out (FIFO) order. Queues are useful for storing a list of tasks that need to be processed in the order they were received, such as a list of print jobs in a printer.
7. A queue might be used to store a list of notifications that need to be shown to the user. Each element in the queue could be an object representing a notification, with information about the type of notification, the sender, and other details. The app could process the notifications in the order they were received, so the user sees them in the correct order.
8. A tree is a data structure that stores a set of elements in a hierarchical structure. Each element in the tree is called a node, and the nodes are connected by edges. Trees are useful for storing data that needs to be organized in a hierarchical way, such as the directory structure of a file system.
9. A tree might be used to store the hierarchical structure of the user's friends on the app. Each node in the tree could represent a person, with edges connecting the node to the person's friends. The tree would allow the app to efficiently search for friends based on their relationships to the user.
21. Linear Data Structures
A linear data structure is a data structure that stores data in a linear or sequential way, meaning that the data is organized in a single line or sequence. Arrays and linked lists are two main types of linear data structures:
example
1. An array is a linear data structure that stores a list of values. Each value in the list is called an element, and the elements can be of different types, such as numbers, strings, or objects. Arrays are useful for storing lists of data that need to be accessed in order, such as a list of high scores in a game.
2. A linked list is a linear data structure that stores a list of elements, with each element pointing to the next element in the list. Linked lists are useful for inserting and deleting elements from the list quickly, because you only need to update the pointers of the elements that come before and after the element you are inserting or deleting.
3. A queue is a linear data structure that stores a list of elements in a first-in, first-out (FIFO) order. Queues are useful for storing a list of tasks that need to be processed in the order they were received, such as a list of print jobs in a printer.
22. Non-Linear Data structure
A non-linear data structure is a data structure that stores data in a way that is not organized in a single line or sequence. There are many different types of non-linear data structures, including trees, graphs, and hash tables.
example
1. A tree might be used in a file browser app to store the hierarchical structure of the user's files and directories. The app could use a tree data structure to store the root directory as the top node, with the subdirectories and files as child nodes connected to the root node by edges. This would allow the app to efficiently display the directory structure and allow the user to navigate through their files.
2. A graph might be used in a social networking app to store the connections between users and the relationships between them. The app could use a graph data structure to store each user as a node, with edges connecting the nodes to represent the relationships between the users. This would allow the app to efficiently find users based on their connections to other users.
3. A hash table might be used in a password manager app to store and look up user passwords quickly. The app could use a hash table to store the passwords as key-value pairs, with the user's login name as the key and the password as the value. The app could use a hash function to map the login names to specific indices in the hash table, allowing it to look up the passwords in constant time.
23. Linked Lists
A linked list is a data structure that stores a list of elements, with each element pointing to the next element in the list. Linked lists are a type of linear data structure, meaning that the elements are organized in a single line or sequence.
example
1. Imagine you have a program that keeps track of a list of songs that you like to listen to. You could create a linked list called "favoriteSongs" that stores the songs in the order you like to listen to them. Each element in the list could be an object representing a song, with information about the song's title, artist, and other details. The linked list would allow you to efficiently insert and delete songs from the list as your tastes change.
2. Let's say, you have a program that helps you plan your day. You could create a linked list called "tasks" that stores a list of things you need to do in the order you want to do them. Each element in the list could be an object representing a task, with information about the task's description, priority, and other details. The linked list would allow you to efficiently insert and delete tasks as your schedule changes.
3. For suppose, you have a program that helps you keep track of your favorite recipes. You could create a linked list called "favoriteRecipes" that stores a list of recipes you like to cook. Each element in the list could be an object representing a recipe, with information about the recipe's name, ingredients, and instructions. The linked list would allow you to efficiently insert and delete recipes as your tastes change.
24. Internet
The Internet is a global network of computers that allows people to communicate and share information with each other. It consists of many different computer systems that are connected to each other using a variety of technologies, such as cables, satellites, and wireless connections.
example
1. Imagine you have a program that allows you to send and receive email messages. The program could use the Internet to connect to an email server, which is a computer that stores and sends email messages. When you send an email, the program would use the Internet to send the message to the email server, which would then forward the message to the recipient's email server. When you receive an email, the program would use the Internet to connect to your email server and download the message.Imagine you have a program that allows you to send and receive email messages. The program could use the Internet to connect to an email server, which is a computer that stores and sends email messages. When you send an email, the program would use the Internet to send the message to the email server, which would then forward the message to the recipient's email server. When you receive an email, the program would use the Internet to connect to your email server and download the message.
2. Imagine you have a program that helps you browse the web. The program could use the Internet to connect to web servers, which are computers that store websites. When you enter a web address into the program, it would use the Internet to send a request to the web server to download the website. The web server would then send the website back to the program, which would display the website on your computer.
3. Imagine you have a program that allows you to make video calls with your friends. The program could use the Internet to connect to a video call server, which is a computer that routes video call traffic between different users. When you make a video call, the program would use the Internet to send a request to the video call server to connect to your friend's computer. The video call server would then establish a connection between your computer and your friend's computer, allowing you to see and hear each other in real-time.
25. Front End
The Front-end refers to the parts of a website or application that the user interacts with directly. It includes the design, layout, and user interface of the app, as well as the client-side code that runs in the user's web browser or app.
The main languages used for front-end development are HTML, CSS, and JavaScript.
We have tutorials for them. Check it here HTML CSS JavaScript
HTML
Hypertext Markup Language (HTML) is a standard for structuring and formatting the content of a website. It is used to define the structure of a webpage and how it should be displayed in a web browser. HTML consists of a series of elements and tags that are used to define the structure and content of a webpage. These elements and tags can be used to create headings, paragraphs, lists, links, and other types of content, as well as to specify the layout and design of the webpage. HTML is an essential part of the World Wide Web and is used to create the vast majority of websites.
HTML is like the blueprint for a house. It defines the structure and layout of the house, including the location of the rooms, windows, and doors. Just as a blueprint is used to guide the construction of a house, HTML is used to define the structure and layout of a web page.
CSS
CSS is a language used to define the style and layout of a webpage written in HTML. It allows web developers to control the appearance of a website by specifying formatting such as font size, color, and layout in a separate file from the HTML content. By separating the content of a webpage from its presentation, CSS makes it easier to maintain and update the look of a website. It is an important tool for web development and is used to style the structure and content of a webpage.
CSS is like the interior design of a house. It defines the look and feel of the house, including the colors, textures, and finishes of the walls, floors, and furniture. Just as interior design is used to make a house look attractive and inviting, CSS is used to style and format a web page to make it look appealing and easy to use.
JavaScript
JavaScript is a programming language that is used to add interactivity and dynamic behavior to a website. It is used to create effects such as animations, form validation, and interactive maps.
JavaScript is like the electrical and plumbing systems of a house. It adds functionality to the house, such as lighting, heating, and water. Just as these systems make a house livable and functional, JavaScript adds interactivity and dynamic behavior to a web page, making it more interactive and engaging for the user.
example
Imagine you have a program that allows you to create and edit documents online. The front-end of the program might use HTML to structure the content of the document, CSS to control the layout and formatting of the document, and JavaScript to add interactive features such as spell check and auto-save.
26. Back End
The Back-end refers to the parts of a website or application that run on the server, rather than on the user's device. It includes the server-side code that runs on the server, as well as the databases that store the data used by the app.
The main languages used for back-end development are server-side languages such as PHP, Python, Node js, Java and Ruby. These languages are used to write the code that runs on the server, handling tasks such as storing and retrieving data from a database, processing user requests, and generating HTML, CSS, and JavaScript that is sent to the user's web browser or app.
Back-end development is used to create the logic and functionality of a website or app, while front-end development is used to create the user interface and design. The front-end and back-end of a website or app are connected through APIs (Application Programming Interfaces), which are sets of rules and protocols that allow the front-end and back-end to communicate with each other.
example
1. Imagine you have a program that allows you to shop online. The back-end of the program might use a server-side language such as PHP to process the user's orders and store them in a database. It might also use a database management system such as MySQL to store information about the user's account, the items in their shopping cart, and their order history. The front-end of the program, which runs on the user's device, might use HTML, CSS, and JavaScript to display the user interface and handle tasks such as displaying the catalog of items, adding items to the cart, and processing the payment.
2.Let's say you have a program that allows you to search for and book flights. The back-end of the program might use a server-side language such as Python to search for flights based on the user's criteria and store the results in a database. It might also use a database management system such as MongoDB to store information about the available flights, the user's search history, and the user's bookings. The front-end of the program might use HTML, CSS, and JavaScript to display the user interface and handle tasks such as displaying the search
27. Libraries
A library is a collection of pre-written code that can be used to perform common tasks in a program. Libraries are a useful tool for programmers because they allow them to reuse code that has already been tested and debugged, rather than having to write the code from scratch. This can save a lot of time and effort, and help ensure that the code is reliable and efficient.
Imagine you have a bunch of building blocks and you want to build a tower. The blocks are like a code library. You can choose the specific blocks that you need to build your tower (your code).
Libraries are typically written in the same language as the program, and they can be used in a variety of contexts, such as web development, data analysis, and machine learning. Some examples of popular libraries include NumPy (a library for scientific computing with Python), pandas (a library for data manipulation and analysis with Python), and TensorFlow (a library for machine learning with Python).
Here is an example that illustrates the difference between writing complete code using a programming language and using a library
Imagine you have a program that displays a list of images on a web page. You want to add a feature that allows the user to filter the images by category. Without using a library, you might write the following code in JavaScript:
const images = [
{ src: 'image1.jpg', category: 'landscape' },
{ src: 'image2.jpg', category: 'portrait' },
{ src: 'image3.jpg', category: 'landscape' },
{ src: 'image4.jpg', category: 'portrait' }
];
function filterImages(category) {
return images.filter(image => image.category === category);
}
const filteredImages = filterImages('landscape');
This code defines an array of images and a function called "filterImages" that takes a category as an argument and returns a new array of images that match the specified category.
Now imagine you want to use a library to filter the images. You could use the Lodash library, which provides a function called "filter" that filters an array based on a given predicate. To use this function, you would first have to import the Lodash library, like this:
import _ from 'lodash';
const images = [
{ src: 'image1.jpg', category: 'landscape' },
{ src: 'image2.jpg', category: 'portrait' },
{ src: 'image3.jpg', category: 'landscape' },
{ src: 'image4.jpg', category: 'portrait' }
];
const filteredImages = _.filter(images, { category: 'landscape' });
This code imports the Lodash library , so that you can use it in your code by calling ".filter". The "filteredImages" variable now contains an array of images that match the "landscape" category.
Advantages of using libraries
1. Time-saving: Libraries allow you to reuse code that has already been written and tested, saving you the time and effort of writing the code yourself.
2.Reliability: Libraries are usually well-documented and well-tested, so you can be confident that they will work correctly in your program.
3. Maintenance: If you use a library in your program, you don't have to worry about maintaining the code yourself.
4. Community support: Many libraries have a large community of users and developers, which can be a useful resource for getting help and support.
5. Code reuse: Libraries allow you to reuse code across multiple projects, saving you the time and effort of writing similar code multiple times.
28. Frameworks
A framework is a set of libraries and tools that provide a structure for building and organizing a software application. Frameworks are designed to make it easier to develop applications by providing a set of standardized components and conventions for building and organizing the code.
Imagine you want to build a house. You could start from scratch and design and build everything yourself, but that would be a lot of work. Instead, you might use a framework, like a set of pre-made blueprints. The blueprints give you a structure to follow and tell you how to build the different parts of the house. You can customize and add your own touches, but you have to work within the structure provided by the blueprints.
Frameworks are often used in conjunction with a programming language, such as JavaScript or Python. They provide a set of libraries and tools that are specific to a particular domain, such as web development or machine learning, and they often include features such as routing, database integration, and user authentication.
Imagine you are building a web application that allows users to create and share notes online. You want to use the Django framework, which is a popular framework for building web applications with Python.
To use Django, you would first install it on your computer and create a new Django project using the Django command-line tools. This would create a basic skeleton of your web application, including directories for your code, templates, and static files.
Next, you would create a Django "app" within your project to hold the code for your notes feature. An app is a self-contained unit of code that performs a specific task within a Django project.
To create the notes feature, you would write code to define a Django "model" that represents the data for a note (such as the title, content, and date). You would also write code to define a Django "view" that handles the logic for displaying and processing the notes (such as creating a new note, editing an existing note, or deleting a note). Finally, you would write code to define a Django "template" that defines the HTML layout and design of your notes pages.
Once you have written this code, you can use the Django command-line tools to "migrate" the database, which will create the necessary tables and fields in the database to store your notes data.
Finally, you would use Django's built-in web server to test your web application locally, and then deploy it to a web server when you are ready to make it available to users.
Difference between Libraries and Frameworks
Libraries and frameworks are both collections of code that provide useful functions and features for developers. However, there are some key differences between the two:
1. Purpose: Libraries are collections of code that you can use to add specific functionality to your own code. For example, the Python "math" library provides functions for performing mathematical operations, like calculating the square root of a number. Frameworks, on the other hand, provide an overall structure for building an application. They usually include both code libraries and other tools, and they often dictate a specific way of organizing and building an application.
2. Inclusion: When you use a library, you usually include the specific pieces of code that you need in your own codebase. With a framework, you build your application using the framework's structure and tools, and you often don't need to include the framework's code directly in your codebase.
3. Control: Because you include specific pieces of code from a library in your own codebase, you have more control over how the library's code is used. With a framework, you have to work within the constraints of the framework and use the tools and structure provided by the framework.
Overall, the main difference between libraries and frameworks is the level of abstraction. Libraries provide specific functionality that you can use in your code, while frameworks provide a higher-level structure for building an application.
29. Server
A server is a computer or computer program that provides a service to other computers or programs. It can be thought of as a kind of "middleman" that sits between the computers that are requesting a service (clients) and the resources that are being provided (such as databases, files, or applications).
Imagine you are at a restaurant and you want to order some food. You (the client) tell the server (the server computer) what you want to eat. The server then goes to the kitchen (the resource) and tells the chef (another program) to prepare your food. The chef gets all the ingredients and cooks your food, then gives it to the server to bring back to you. The server brings the food (the response) back to you, and you can eat it.
Now, let's look at how servers work in the context of an online application like Instagram or Facebook:
When you open the Instagram or Facebook app on your phone, you are making a request to the server to see your feed or profile. The server receives your request and goes to the database (a resource) to retrieve the information you requested. The server then sends the information back to your phone (the response), and you can see your feed or profile on the app.
In this example, your phone is the client, the server is the server computer, and the database is the resource. The server acts as a middleman, communicating with the client (your phone) and the resource (the database) to fulfill the request (showing your feed or profile).
30. Data Bases
A database is a collection of information that is stored electronically and can be easily accessed and modified. Databases are used to store and organize large amounts of data, and they are used in many different types of applications, including online stores, social media platforms, and customer relationship management systems.
Imagine you have a library full of books. Each book has a title, an author, and a number of pages. You want to be able to find specific books quickly, so you create a list of all the books in the library and the information about each book. This list is like a database. You can use the database to find a specific book by looking for the title, the author, or the number of pages.
Let's look at how databases work in the context of an online application like Instagram or Facebook:
When you post a photo on Instagram or a status update on Facebook, the app stores that information in a database. The database stores the photo or update, along with other information like the time it was posted and your username. When you open the app and want to see your feed or profile, the app retrieves the information from the database and displays it for you.
In this example, the database is like a library of all the photos and updates that have been posted on Instagram or Facebook. The app (the client) can access the database (the resource) to retrieve the information it needs to show you your feed or profile.
31. Cloud
The cloud is a way of storing and accessing data and programs over the Internet, instead of storing them on a computer or server. When you use the cloud, you can access your data and programs from any device with an Internet connection, and you don't have to store anything on your own computer.
Imagine you have a bunch of toys and you want to share them with your friends. You could give each of your friends a toy to keep, but then you wouldn't have any toys left for yourself. Instead, you could put all your toys in a big toy box and let your friends play with the toys when they come over. The toy box is like the cloud. You can access the toys (the data and programs) from anywhere, and you don't have to store them on your own shelf (your computer).
32. Difference between Cloud and Data Base
A cloud and a database are both ways of storing and accessing data electronically, but they are used for different purposes and have some key differences.
Here's an example to help explain the difference between a cloud and a database:
1. Purpose: A database is used to store and organize data in a structured way, and it is designed to make it easy to retrieve and use specific pieces of data. A cloud is a way of storing and accessing data and programs over the Internet, and it is designed to make it easy to access data and programs from any device with an Internet connection.
2. Accessibility: A database is typically stored on a single computer or server, and it can only be accessed by other computers or programs that are connected to that server. A cloud is a network of servers that are connected to the Internet, and it can be accessed from anywhere with an Internet connection.
3. Control: With a database, you usually have more control over the data and how it is used. You can choose which data to store and how to structure it, and you can set up permissions to control who can access and modify the data. With a cloud, you have less control over the data and how it is used. You have to work within the constraints of the cloud provider's services and terms of use.
4. Cost: Using a database usually requires upfront costs for hardware, software, and maintenance. A cloud can be more cost-effective because you only pay for the resources you use, and you don't have to worry about maintaining the hardware and software yourself.
Imagine you have a bunch of toys and you want to share them with your friends. You could put all your toys in a big toy box and let your friends play with the toys when they come over. The toy box is like the cloud. You can access the toys (the data and programs) from anywhere, and you don't have to store them on your own shelf (your computer).
Now, imagine you want to keep track of which toys you have and which toys your friends have. You could create a list of all the toys and mark which toys are yours and which toys belong to your friends. This list is like a database. You can use the database to find a specific toy by looking for its name or type, and you can see which toys are yours and which toys belong to your friends.
Now, let's look at how a cloud and a database might be used in a real-world example, like a social media app:
When you use a social media app like Instagram or Facebook, the app stores all the photos, updates, and other data in a database. The database stores the data, along with other information like the time it was posted and your username. When you open the app and want to see your feed or profile, the app retrieves the data from the database and displays it for you.
The social media app also uses the cloud to store the data. The data is stored on servers (large computers) in data centers, which are connected to the Internet. This means you can use the app on your phone, tablet, or computer to access your data and use the app, without having to store anything on your own device.
In this example, the database is like the list of toys, and the cloud is like the toy box. The database is used to store and organize the data (the toys) and make it easy to retrieve and use. The cloud is used to store the data (the toys) and make it accessible from anywhere with an Internet connection.
33. How Computer Works?
At a high level, a computer works by using a processor (a kind of "brain") to execute instructions that tell the computer what to do. These instructions are called programs or code, and they are written by programmers using a programming language.
1. Input: The first step in the process is to input data into the computer. This can be done in a variety of ways, such as typing on a keyboard, clicking a mouse, or scanning a barcode.
2. Storage: The data that is input into the computer needs to be stored somewhere so it can be accessed and used later. The computer stores the data in its memory (such as the RAM or hard drive).
3. Processing: Once the data is stored in the computer's memory, the processor (the "brain" of the computer) can access the data and execute instructions (the code) to perform a task. The processor reads the instructions one by one and executes them in order.
4. Output: After the processor has executed the instructions, the computer produces an output. This could be a result of a calculation, a message on the screen, or a printed document.
5. Communication: A computer can also communicate with other devices and computers over a network (such as the Internet). This allows it to send and receive data and instructions to and from other devices.
example
Imagine you have a recipe for a cake and you want to use your computer to help you make the cake. You input the recipe into the computer by typing it into a word processor (such as Microsoft Word). The computer stores the recipe in its memory.
Next, you use a program (such as a calculator) to help you convert the recipe's measurements from cups to grams. You input the measurement (1 cup of sugar) into the calculator program, and the program uses its instructions (the code) to perform a calculation (1 cup of sugar is equal to 200 grams). The program outputs the result (200 grams) on the screen.
Finally, you use the computer to communicate with a printer to print out the recipe so you can take it to the kitchen. The computer sends the recipe to the printer, and the printer produces a printed copy of the recipe.
In this example, the input is the recipe that you typed into the word processor, the storage is the computer's memory where the recipe is stored, the processing is the calculation performed by the calculator program, the output is the result displayed on the screen, and the communication is the sending of the recipe to the printer.
34. Pixels
Pixels are the smallest units of a digital image or display. They are tiny dots of color that, when combined, create the overall image or display.
In a digital image or display, the pixels are arranged in a grid pattern, with each pixel having a specific position on the grid. The pixels are so small that they are not visible to the naked eye, but when they are combined, they create the overall image or display.
The number of pixels in an image or display is often used to determine the size and quality of the image or display. The more pixels there are in an image or display, the higher the resolution and the clearer the image will be.
For example, a digital image with a resolution of 1920 x 1080 pixels (1080p) will be higher quality and have more detail than an image with 1280 x 720 pixels (720p). Similarly, a display with a higher resolution (such as 4K or 8K) will be able to show more detail and provide a higher quality image than a display with a lower resolution (such as 1080p).
The size of an image or display is often measured in pixels. For example, a digital image that is 1920 pixels wide and 1080 pixels tall (also known as 1080p) will be larger than an image that is 1280 pixels wide and 720 pixels tall (also known as 720p). Similarly, a display with a higher resolution (such as 4K or 8K) will be able to show a larger image than a display with a lower resolution (such as 1080p).
In video games, the number of pixels and the resolution of the display can have a significant impact on the quality and performance of the game. Higher resolution displays can provide more detailed and realistic graphics, but they can also require more processing power to run smoothly.
example
Imagine you have a puzzle with lots of tiny puzzle pieces. Each puzzle piece is a pixel. When you put all the puzzle pieces together, they create a picture. The pixels in a digital image or display work in the same way. When you put all the pixels together, they create the overall image or display.
n a digital image or display, the pixels are arranged in a grid pattern, with each pixel having a specific position on the grid. The pixels are so small that they are not visible to the naked eye, but when they are combined, they create the overall image or display.
Pixels are important because they determine the resolution of an image or display. The more pixels there are in an image or display, the higher the resolution and the clearer the image will be.
35. Video Games
Video games are created using a variety of programming languages and frameworks. The specific languages and frameworks used can vary depending on the game and the platform it is being developed for (such as a PC, a console, or a mobile device).
Some common programming languages and frameworks used in game development include:
1. C++: C++ is a high-performance programming language that is often used in game development. It is a good choice for creating fast, resource-intensive games.
2. C#: C# is a programming language that is designed for building Windows applications. It is often used in game development because it is easy to learn and use, and it has a wide range of tools and libraries available.
3. Unity: Unity is a cross-platform game engine that is used to develop games for a variety of platforms, including PC, console, and mobile. It includes tools for creating graphics, sound, and gameplay, and it supports a range of programming languages, including C# and UnityScript (a variant of JavaScript).
4. Unreal Engine: Unreal Engine is a game engine developed by Epic Games. It is used to create high-quality games for a variety of platforms, including PC, console, and mobile. It includes tools for creating graphics, sound, and gameplay, and it supports a range of programming languages, including C++ and Blueprints (a visual scripting language).
Thank You
Thank you for visiting our site to learn about computer programming fundamentals and the different programming languages. We hope that you found the information helpful and that it has given you a good foundation for your journey in programming.
We encourage you to revisit our site as you continue to learn and grow in your skills. We have many other tutorials available that build upon the basic concepts you have learned, and we recommend starting with these before moving on to more advanced topics.
Thank you again for choosing WebDevMonk as a resource for your learning. We hope to see you back here soon!