C++ Basics Tutorial
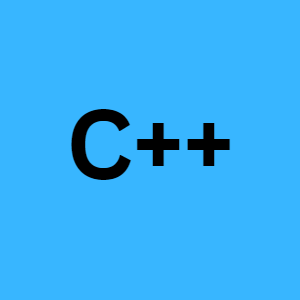
Learn the fundamentals of programming with our tutorial The Building Blocks of Computer Programming Beginner-friendly with essential concepts and interactive exercises designed to aid learning and retention
Concepts:
11. C++ if, if-else, switch statements
12. C++ while, do-while, for loops
1. C++ Introduction
C++ is a high-performance programming language that is widely used in a variety of fields, including operating systems, web browsers, and various applications. C++ is an extension of the C language, which was developed in the 1970s.
C++ is an object-oriented programming language, which means that it is designed to support the creation and manipulation of "objects" that represent real-world entities.
Why to learn C++?
1. C++ is widely used. Many companies use C++ in their software development, so learning the language can make you a more attractive candidate for a job in the tech industry.
2. C++ is a general-purpose programming language that is widely used in a variety of fields.
3. Operating systems: C++ is often used to develop the core components of operating systems, such as the kernel and device drivers.
4. Web browsers: Many web browsers, such as Google Chrome and Mozilla Firefox, are written in C++.
5. Applications: C++ is used to develop a wide range of applications, including office suites, graphic design software, and games (GTA 5, Fortnite, Dota 2, World of Warcraft).
2. C++ Syntax
A common structure for a C++ program includes the following elements
1.Preprocessor directives:Preprocessor directives are lines of code that are processed by a preprocessor before the source code is compiled. They are typically used to include header files, define macros, and perform other tasks that need to be done before the code is compiled.
2. Function prototypes: These are declarations of functions that are used in the program. They include the function name, the data types of the parameters, and the return type.
3. Global variables: These are variables that are declared outside of any function and are available to all functions in the program.
4. main() function: This is the entry point of the program and is where the program's execution begins. It is typically used to call other functions and perform tasks.
5. other functions: These are functions that are defined and used in the program. They can be called from the main() function or from other functions.
#include <iostream>
void greet(); // function prototype
int main() {
greet();
return 0;
}
void greet() {
std::cout << "Hello, World!" << std::endl; // Hello, World!
}
3. C++ Output
In C++, you can use the 'std::cout' object to output text to the console. The 'std::cout' object is part of the iostream library, which is included using the '#include <iostream>' directive at the top of the program.
To output text using std::cout, you can use the << operator, followed by the text you want to output
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Hello, World!
return 0;
}
This will output the text "Hello, World!" to the console. The 'std::endl' inserts a newline character after the text is output.
4. C++ Sum of Two Numbers
#include <iostream>
int main() {
int a = 10;
int b = 20;
int sum = a + b;
std::cout << "The sum of a and b is: " << sum << std::endl; // The sum of a and b is: 30
return 0;
}
This will output the text "The value of x is: 30" to the console.
5. C++ Comments
Comments are used in C++ to add notes and explanations to the code. They are ignored by the compiler and are used only to help the programmer understand the code.
There are two types of comments in C++:
1. single-line comments
2. multi-line comments.
Single-line comments start with // and continue until the end of the line.
int a = 10; // Declare and initialize variable x
Multi-line comments start with /* and end with */.
/*
This is a multi-line
comment. It can span
multiple lines.
*/
You can also use comments to "comment out" a block of code that you don't want to execute.
/*
int a = 10;
int b = 20;
int sum = a + b;
std::cout << "The sum is: " << sum << std::endl;
*/
In this example, the code between the /* and */ will not be executed, but it will still be present in the program. This can be useful for testing or debugging.
6. C++ Variables
In C++, a variable is a named location in memory where you can store a value. Variables must be declared with a specific data type, such as 'int' for integers or 'char' for characters.
Here is an example of how to declare and initialize a variable in C++
int A = 10; // A and a are not same
char c = 'A';
float f = 3.14;
// You can also declare multiple variables of the same type on a single line, like this:
int th= 10, second_num = 20, c = 30;
declaring a variable without initializing it is also allowed
int a;
In this case, the value of the variable is undefined until it is assigned a value later in the program.
It is important to choose descriptive names for your variables to make your code easier to read and understand. Variable names can contain letters, digits, and the underscore character '(_)', but they must not begin with a digit.
7. C++ Input
In C++, you can use the 'std::cin' object to read input from the console. The 'std::cin' object is part of the iostream library, which is included using the '#include <iostream>' directive at the top of the program.
To read input using 'std::cin', you can use the >> operator, followed by the variable you want to store the input in.
#include <iostream>
int main() {
int x;
std::cout << "Enter a number: ";
std::cin >> x;
std::cout << "You entered: " << x << std::endl;
return 0;
}
/*
Output :
Enter a number: 2
You entered: 2
*/
This will output the text "Enter a number: " to the console and wait for the user to enter a number. The entered number will be stored in the x variable, and then the program will output the text "You entered: [number]" to the console.
8. C++ Data Types
In C++, data types refer to the type and size of data that a variable or a constant can hold. There are several built-in data types in C++, which include:
char:
The 'char' data type in C++ is used to hold character values. It occupies 1 byte of memory and can hold any character from the ASCII table, which includes alphabets (both uppercase and lowercase), digits, and special characters.
int:
This 'int' data in C++ type is used to store the integer values. It occupies 2 or 4 bytes of memory, depending on the system, and can hold whole numbers within a certain range.
float:
This 'float' type in C++ is used to store floating-point values of variables. It occupies 4 bytes of memory and can hold decimal values with a certain degree of precision.
double:
This data type in C++ is also used to store floating-point values. It occupies 8 bytes of memory and can hold decimal values with a higher degree of precision than float.
bool:
This data type in C++ is used to store boolean values, which can be either true or false. It occupies 1 byte of memory.
#include <iostream>
int main()
{
// Declare teh variables of several data types
char c = 'A';
int i = 10;
float f = 3.14;
double d = 123.456;
bool b = true;
// Print the values of the variables
std::cout << "c = " << c << std::endl;
std::cout << "i = " << i << std::endl;
std::cout << "f = " << f << std::endl;
std::cout << "d = " << d << std::endl;
std::cout << "b = " << b << std::endl;
return 0;
}
// Output:
/*
c = A
i = 10
f = 3.14
d = 123.456
b = 1
*/
9. C++ Booleans
In C++, boolean values are represented using the bool data type. A boolean value can be either 'true' or 'false', and it occupies 1 byte of memory.
For 'true' the value is 1 and for 'false' the value is 0
#include <iostream>
int main()
{
bool flag = true;
if (flag)
{
std::cout << "flag is true" << std::endl; // flag is true
}
else
{
std::cout << "flag is false" << std::endl;
}
return 0;
}
Boolean values are often used in conditional statements, such as 'if', 'while', and 'for', to control the flow of a program.
10. C++ Operators
C++ operators are the kind symbols that perform specific operations on one or more operands (values or variables).
1. Arithmetic Operators
The arithmetic operators are used to perform basic mathematical operations on variables and values.
1. ' + ' : a+b
2. ' - ' : a-b
3. ' * ' : a*b
4. ' / ' : a/b
5. ' % ' : a%b (returns the remainder when a is divided by b.)
#include <iostream>
int main() {
int a = 10;
int b = 5;
std::cout << a + b << std::endl; // Outputs 15
std::cout << a - b << std::endl; // Outputs 5
std::cout << a * b << std::endl; // Outputs 50
std::cout << a / b << std::endl; // Outputs 2
std::cout << a % b << std::endl; // Outputs 0
return 0;
}
2. Assignment Operators
In C++, there are several assignment operators that can be used to assign a value to a variable
1. ' = ' : a = 9
2. ' += ' : a += 9 (a = a+9)
3. ' -= ' : a-=9
4. ' *= ' : a*=9
5. ' /= ' : a/=9
6. ' %= ' : a%=9
7. ' >>= ' : a>>=9
8. ' <<= ' : a<<=9
9. ' ^= ' : a^=9
9. ' &= ' : a&=9
10. ' |= ' : a|=9
#include <iostream>
int main()
{
int a = 10;
int b = 5;
std::cout << "a = " << a << std::endl;
std::cout << "b = " << b << std::endl;
a += b; // a is now 15
std::cout << "a += b: a = " << a << std::endl;
a -= b; // a is now 10
std::cout << "a -= b: a = " << a << std::endl;
a *= b; // a is now 50
std::cout << "a *= b: a = " << a << std::endl;
a /= b; // a is now 10
std::cout << "a /= b: a = " << a << std::endl;
a %= b; // a is now 0
std::cout << "a %= b: a = " << a << std::endl;
a <<= 1; // a is now 0
std::cout << "a <<= 1: a = " << a << std::endl;
a >>= 1; // a is now 0
std::cout << "a >>= 1: a = " << a << std::endl;
a &= b; // a is now 0
std::cout << "a &= b: a = " << a << std::endl;
a ^= b; // a is now 5
std::cout << "a ^= b: a = " << b << std::endl;
a |= b; // a is now 5
std::cout << "a |= b: a = " << a << std::endl;
return 0;
}
// Output:
/*
a = 10
b = 5
a += b: a = 15
a -= b: a = 10
a *= b: a = 50
a /= b: a = 10
a %= b: a = 0
a <<= 1: a = 0
a >>= 1: a = 0
a &= b: a = 0
a ^= b: a = 5
a |= b: a = 5
*/
3. Comparision Operators
1. ' == ' : a==b
2. ' < ': a<b
3. ' > ' : a>b
4. ' <= ' : a<=b
5. ' >= ' : a>=b
6. ' != ' : a!=b
#include <iostream>
int main()
{
int a = 10;
int b = 5;
std::cout << "a = " << a << std::endl;
std::cout << "b = " << a << std::endl;
// Equal to
std::cout << "a == b: " << (a == b) << std::endl; // False
// Not equal to
std::cout << "a != b: " << (a != b) << std::endl; // True
// Greater than
std::cout << "a > b: " << (a > b) << std::endl; // True
// Less than
std::cout << "a < b: " << (a < b) << std::endl; // False
// Greater than or equal to
std::cout << "a >= b: " << (a >= b) << std::endl; // True
// Less than or equal to
std::cout << "a <= b: " << (a <= b) << std::endl; // False
return 0;
}
// Output:
/*
a = 10
b = 5
a == b: 0
b != b: 1
a > b: 1
a < b: 0
a >= b: 1
a <= b: 0
*/
4. Logical Operators
1. ' && ' : x < 5 && x < 10 (return 'true' if both conditions are true)
2. ' || ' : x < 5 || x < 4 (return 'true' if any conditions are true)
3. ' ! ' : !(x < 5 && x < 10) (Reverse the result, returns 'false' even the result is 'true')
#include <iostream>
int main()
{
bool a = true;
bool b = false;
std::cout << "a = " << a << std::endl;
std::cout << "b = " << b << std::endl;
// Logical AND
std::cout << "a && b: " << (a && b) << std::endl; // False
// Logical OR
std::cout << "a || b: " << (a || b) << std::endl; // True
// Logical NOT
std::cout << "!a: " << (!a) << std::endl; // False
std::cout << "!b: " << (!b) << std::endl; // True
return 0;
}
// Output:
/*
a = 1
b = 0
a && b: 0
a || b: 1
!a: 0
!b: 1
*/
5. Increment and Decrement operators
1. ' ++ ' : a++ or ++a (a = a+1)
2. ' -- ' : a-- or --a (a = a-1)
#include <iostream>
int main()
{
int a = 0;
a++; // add 1 to a (a is now 1)
std::cout << a << std::endl;
++a; // add 1 to a (a is now 2)
std::cout << a << std::endl;
a--; // subtract 1 from a (a is now 1)
std::cout << a << std::endl;
--a; // subtract 1 from a (a is now 0)
std::cout << a << std::endl;
return 0;
}
// Output:
/*
1
2
1
0
*/
6. Conditional Operators
In C++, the conditional operator (also known as the ternary operator) is a way to perform a conditional expression.
// syntax
condition ? expression1 : expression2
#include <iostream>
int main()
{
int a = 10;
int b = 20;
int c = (a > b) ? a : b; // if a is greater than b, c will be set to a; otherwise, c will be set to a
std::cout << "c: " << c << std::endl;
return 0;
}
// Output:
// z: 20
7. Bitwise operators
In C++, bitwise operators are used to perform bit-level operations on variables.
1. ' & ' : (bitwise AND)
2. ' | ' : (bitwise OR)
3. ' ^ ' : (bitwise XOR)
4. ' ~ ' : (bitwise NOT)
5. ' << ' : (left shift)
6. ' >> ' : (right shift)
#include <iostream>
int main()
{
int a = 10; // binary representation: 1010
int y = 7; // binary representation: 0111
std::cout << (a & b) << std::endl; // bitwise AND: 0010 (2)
std::cout << (a | b) << std::endl; // bitwise OR: 1111 (15)
std::cout << (a ^ b) << std::endl; // bitwise XOR: 1101 (13)
std::cout << (~a) << std::endl; // bitwise NOT: 0101 (-11)
std::cout << (a << 1) << std::endl; // left shift: 10100 (20)
std::cout << (b >> 1) << std::endl; // right shift: 0011 (3)
return 0;
}
// Output:
/*
2
15
13
-11
20
3
*/
Note that the bitwise NOT operator '(~)' inverts the bits of a number, while the left shift ('<<)' and right shift '(>>)' operators shift the bits of a number to the left or right, respectively. The number of positions to shift the bits is specified as an operand.
8. Other operators
1. Sizeof operator: The sizeof operator returns the size, in bytes, of a variable or data type.
#include <iostream>
int main()
{
int i = 10;
float f = 3.14;
double d = 3.14159265358979323846;
std::cout << "Size of int: " << sizeof(i) << " bytes" << std::endl;
std::cout << "Size of float: " << sizeof(f) << " bytes" << std::endl;
std::cout << "Size of double: " << sizeof(d) << " bytes" << std::endl;
return 0;
}
// Output:
/*
Size of int: 4 bytes
Size of float: 4 bytes
Size of double: 8 bytes
*/
2. Member access operators: These operators are used to access members of a class or struct. The dot operator (.) is used to access a member of an object, while the arrow operator (->) is used to access a member of a pointer to an object.
#include <iostream>
class Point
{
public:
int a, b;
Point(int a = 0, int b = 0)
: a(a), b(b)
{
}
};
int main()
{
Point p1(1, 2);
Point *p2 = new Point(3, 4);
std::cout << "p1.a: " << p1.a << std::endl;
std::cout << "p1.b: " << p1.b << std::endl;
std::cout << "p2->a: " << p2->a << std::endl;
std::cout << "p2->b: " << p2->b << std::endl;
delete p2;
return 0;
}
// Output:
/*
p1.a: 1
p1.b: 2
p2->a: 3
p2->b: 4
*/
11. C++ if, if-else, switch statements
if
In C++, the 'if' statement allows you to specify a block of code to be executed if a certain condition is met(true). The syntax for an 'if' statement is as follows:
if (condition) {
// execute if condition is true
}
int a = 5;
if (a > 4) {
std::cout << "a is greater than 4" << std::endl; // a is greater than 4
}
The above example C++ program, will output 'a is greater than 4' because the condition x > 4 is true.
if-else
The 'if-else' statement is an extension of the if statement that allows you to specify a block of code to be executed if the condition is 'true', and a different block of code is used to be executed if the condition is 'false'. The syntax for an 'if-else' statement is as follows:
if (condition) {
// execute if condition is true
} else {
// execute if condition is false
}
int a = 5;
if (a > 4) {
std::cout << "a is greater than 4" << std::endl; // a is greater than 4
} else {
std::cout << "a is not greater than 4" << std::endl;
}
This will output 'a is greater than 4' because the condition a > 4 is true. If not 'a is not greater than 4' will print
switch
The 'switch' statement is another way to specify a block of code to be executed based on a condition. It allows you to specify a number of different cases, and the code associated with each case will be executed if the condition matches the case. The syntax for a switch statement is as follows:
switch (expression) {
case value1:
// execute if expression == value1
break;
case value2:
// execute if expression == value2
break;
...
default:
// if expression does not match any of the cases, this block will execute
}
int a = 5;
switch (a) {
case 1:
std::cout << "a is 1" << std::endl;
break;
case 2:
std::cout << "a is 2" << std::endl;
break;
case 5:
std::cout << "a is 5" << std::endl; // a is 5
break;
default:
std::cout << "a is not 1 or 5" << std::endl;
}
This will output 'a is 5' because the value of a matches the case 5.
It's important to note that the 'break' statement is used to exit the switch statement and prevent the code from falling through to the next case. If you omit the 'break' statement, the code will continue to execute through all the remaining cases until it reaches the end of the switch statement or a 'break' statement is encountered.
12. C++ while, do-while, for loops
while loop
In C++, the while loop allows you to execute a block of code repeatedly as long as a specific condition is true. The syntax is:
while (condition) {
// statements to execute as long as the condition is true
}
int a = 0;
while (a < 5) {
std::cout << a << std::endl;
a++;
}
/*
Output:
0
1
2
3
4
*/
This will output the numbers 0 through 4 because the condition x < 5 is checked at the beginning of each iteration and the loop will continue as long as the condition is true.
do-while
The 'do-while' loop is similar to the 'while' loop, but the condition is checked at the end of each iteration instead of at the beginning. This means that the code in the loop will always be executed at least once, regardless of the value of the condition. The syntax for a 'do-while' loop is as follows:
do {
// execute at least once
} while (condition);
int a = 9;
do{
std::cout << a << std::endl; // 9
a++;
}while(a>10);
Even though the condition is false it prints 9 to the console that means it execute at least once.
for loop
The 'for' loop is a more concise way to write a loop that performs a specific number of iterations. The syntax for a for loop is as follows:
for (initialization; condition; update) {
// execute as long as condition is true
}
The 'initialization' statement is executed before the loop starts and is usually used to initialize a loop counter. The 'condition' is checked at the beginning of each iteration, and the loop will continue as long as the condition is true. The 'update' statement is executed at the end of each iteration and is usually used to increment or decrement the loop counter.
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
/*
Output:
0
1
2
3
4
*/
This will output the numbers 0 through 4
for (int i = 5; i > 0; i--) {
std::cout << i << std::endl;
}
/*
Output:
5
4
3
2
1
*/
This will output the numbers from 5 through 1
13. C++ break and continue
break
In C++, the break statement is used to exit a loop or a switch statement and transfer control to the statement immediately following the loop or switch.
int a = 0;
while (a < 5) {
std::cout << a << std::endl;
a++;
if (a == 2) {
break;
}
}
/*
Output:
0
1
*/
In the above C++ program, the while loop is said to run from 0 to 4 but because of the 'break' statement, it exit after printing 1
continue
The 'continue' statement is used to skip the rest of the current iteration of a loop and move on to the next iteration.
for (int i = 0; i < 10; i++) {
if (i % 2 == 1) {
continue;
}
std::cout << i << std::endl;
}
/*
Output:
0
2
4
6
8
*/
This will output the numbers 0, 2, 4, 6, 8 because the 'continue' statement is used to skip the current iteration of the loop if the value of i is odd.
14. C++ Arrays
In C++, an array is a collection of elements of the same type stored in contiguous memory locations. Arrays are indexed with integers, and the elements of an array can be accessed using their index.
int arr[5] = {1, 2, 3, 4, 5};
This creates an array of 5 elements, with the elements initialized to the values 1, 2, 3, 4, and 5. The array is indexed from 0 to 4, so the first element of the array is arr[0], the second element is arr[1], and so on.
You can also declare an array without initializing its elements, in which case the elements will be initialized to their default values:
int arr[5]; // elements are initialized to 0
You can access the elements of an array using the array index, for example:
int x = arr[2]; // x is assigned the value 3
You can also modify the elements of an array using the array index
arr[3] = 10; // the fourth element of the array is now 10
To input an array from a user in C++, you can use a loop to prompt the user to enter the values of the array one by one.
#include <iostream>
using namespace std;
const int MAX_SIZE = 100;
int main()
{
int array[MAX_SIZE];
int size;
// to enter the size of the array
cout << "Enter the size of the array: ";
cin >> size;
// to enter the values of the array
for (int i = 0; i < size; i++)
{
cout << "Enter element " << i + 1 << ": ";
cin >> array[i];
}
// Print the array
cout << "The array is: [ ";
for (int i = 0; i < size; i++)
{
cout << array[i] << " ";
}
cout << "]" << endl;
return 0;
}
// Output
/*
Enter the size of the array: 5
Enter element 1: 1
Enter element 2: 2
Enter element 3: 5
Enter element 4: 6
Enter element 5: 4
The array is: [ 1 2 5 6 4 ]
*/
This code will prompt the user to enter the size of the array, and then prompt the user to enter each element of the array one by one. The resulting array will be stored in the array variable.
15. C++ Structures
In C++, a structure is a user-defined data type that can hold a collection of variables of different data types. Structures are often used to represent real-world entities that have multiple attributes, such as a student with a name, an age, and a grade point average.
struct structure_name
{
type variable_name_1;
type variable_name_2;
...
};
To create a variable of this structure type, you can use the following syntax:
student s;
You can then access the individual attributes of the structure using the dot operator '(.)'.
s.name = "John";
s.age = 20;
s.gpa = 3.5;
You can also create an array of structures
student students[MAX_SIZE];
where MAX_SIZE is a constant representing the maximum number of students. You can then access the individual elements of the array using an index, like this:
students[0].name = "John";
students[0].age = 20;
students[0].gpa = 3.5;
students[1].name = "Jane";
students[1].age = 21;
students[1].gpa = 3.6;
You can also define functions that operate on structures.
void print_student(student s)
{
cout << "Name: " << s.name << endl;
cout << "Age: " << s.age << endl;
cout << "GPA: " << s.gpa << endl;
}
You can call this function
student s;
s.name = "John";
s.age = 20;
s.gpa = 3.5;
print_student(s);
#include <iostream>
#include <string>
using namespace std;
// Define the structure for a student
struct student
{
string name;
int age;
float gpa;
};
// Function to print the information for a student
void print_student(student s)
{
cout << "Name: " << s.name << endl;
cout << "Age: " << s.age << endl;
cout << "GPA: " << s.gpa << endl;
}
int main()
{
// Create a student variable
student s;
s.name = "John";
s.age = 20;
s.gpa = 3.5;
// Print the information for the student
print_student(s);
return 0;
}
// Output
/*
Name: John
Age: 20
GPA: 3.5
*/
16. C++ References
In C++, a reference is a way to refer to a variable by an alias, rather than by its actual name. This can be useful when you want to pass a variable to a function by reference, allowing the function to modify the original variable rather than just a copy of it.
int x = 5;
int& y = x; // y is a reference to x
y = 10; // this modifies x
std::cout << x << std::endl; // prints 10
#include <iostream>
void increment(int &x) {
x++;
}
int main() {
int a = 10;
std::cout << "a = " << a << std::endl;
increment(a);
std::cout << "a = " << a << std::endl;
return 0;
}
/*
Output:
a = 10
a = 11
*/
In this example, we have defined a function increment that takes an integer reference as an argument. Inside the function, we increment the value of the reference, which has the effect of incrementing the original value of the variable passed to the function. When we call the function with the variable a, the value of a is incremented from 10 to 11.
References are declared using the '&' symbol, and they are accessed just like regular variables. It is important to note that once a reference is initialized, it cannot be changed to refer to a different object. This is in contrast to pointers, which can be reassigned to point to different objects.
17. C++ Pointers
In C++, a pointer is a variable that stores the address of another variable. Pointers are useful for working with arrays and dynamically allocated memory, and they can also be used to pass large amounts of data to functions more efficiently.
To declare a pointer in C++, you use the * operator in the variable declaration.
int* ptr; // ptr is a pointer to an int
double* dptr; // dptr is a pointer to a double
To use a pointer, you must first initialize it to the address of a variable. This is done using the & operator, which returns the address of a variable.
int a = 5;
int* ptr = &a; // ptr now points to x
*ptr = 10; // this modifies x
std::cout << a << std::endl; // prints 10
In this example, the pointer ptr is initialized to the address of a, and then the value of x is modified by dereferencing ptr and assigning a new value to it using the * operator. This changes the value of a, which is then printed to the console.
#include <iostream>
int main() {
// Declare and initialize an int variable
int x = 5;
// Declare a pointer to an int
int* ptr;
// Initialize the pointer to the address of x
ptr = &x;
// Print the value of x and the address of x
std::cout << "x: " << x << ", &x: " << &x << std::endl;
// Print the value of ptr and the address stored in ptr
std::cout << "ptr: " << ptr << ", *ptr: " << *ptr << std::endl;
// Modify the value of x using the pointer
*ptr = 10;
// Print the new value of x
std::cout << "x: " << x << std::endl;
return 0;
}
// Output:
/*
x: 5, &x: 0x7ffc49b3576c
ptr: 0x7ffc49b3576c, *ptr: 5
x: 10
*/
18. C++ Functions
In C++, a function is a block of reusable code that performs a specific task and may return a value. Functions are useful for organizing and reusing code, and they allow you to break a large program into smaller, more manageable pieces.
To declare a function in C++, you use the 'function_name', 'parameter list (if any)', and return_type (if want to return a value) syntax. For example:
int add(int x, int y) {
return x + y;
}
To call a function in C++, you use the function_name(argument_list)
int result = add(3, 4); // result is 7
In this example, the add function is called with the arguments 3 and 4, and the returned value of 7 is stored in the variable result.
In C++, functions can also be overloaded, which means that you can have multiple functions with the same name but different parameter lists.
double add(double x, double y) {
return x + y;
}
std::string add(std::string x, std::string y) {
return x + y;
}
Now you have two add functions, one that takes two double arguments and returns a double, and another that takes two std::string arguments and returns a std::string. The correct function will be called depending on the type of arguments you pass to it.
#include <iostream>
// Declaring a function that takes two integer(int) arguments and returns an int
int add(int x, int y) {
return x + y;
}
// Declare a function that takes no arguments and returns void
void printHello() {
std::cout << "Hello, world!" << std::endl;
}
int main() {
// Calling the add function and print the result
int result = add(3, 4);
std::cout << "3 + 4 = " << result << std::endl;
// Calling the printHello function
printHello();
return 0;
}
// Output:
/*
3 + 4 = 7
Hello, world!
*/
This program declares two functions: add, which takes two int arguments and returns their sum as an int, and printHello, which takes no arguments and prints "Hello, world!" to the console.
In the main function, the add function is called with the arguments 3 and 4, and the returned value is stored in the variable result. The result variable is then printed to the console.
Next, the printHello function is called, which prints "Hello, world!" to the console.
19. C++ OOP concept
Object-oriented programming (OOP) is a programming model that is based on the concept of "objects", which are data structures that contain both data and functions.
In C++, you can use OOP by defining classes, which are templates for creating objects. A class defines the data and functions that an object will have, as well as the relationships between different objects.
class Dog {
public:
// Constructor
Dog(std::string name, int age) : name_(name), age_(age) {}
// Member functions
void bark() { std::cout << "Woof!" << std::endl; }
void setAge(int age) { age_ = age; }
std::string getName() { return name_; }
int getAge() { return age_; }
private:
// Data members
std::string name_;
int age_;
};
This class defines a Dog object with a name and age, and it has four member functions: a constructor, which is used to create a new Dog object; bark, which prints "Woof!" to the console; setAge, which sets the age of the Dog; and getName and getAge, which return the name and age of the Dog, respectively.
To create a new Dog object, you use the new operator and call the constructor:
Dog* dog1 = new Dog("Max", 3);
You can then call the member functions of the Dog object using the (.) operator
dog1->bark(); // prints "Woof!"
dog1->setAge(4);
std::cout << dog1->getName() << " is " << dog1->getAge() << " years old." << std::endl; // prints "Max is 4 years old."
#include <iostream>
// Define a Dog class
class Dog {
public:
// Constructor
Dog(std::string name, int age) : name_(name), age_(age) {}
// Member functions
void bark() { std::cout << "Woof!" << std::endl; }
void setAge(int age) { age_ = age; }
std::string getName() { return name_; }
int getAge() { return age_; }
private:
// Data members
std::string name_;
int age_;
};
int main() {
// Create three Dog objects
Dog* dog1 = new Dog("Max", 3);
Dog* dog2 = new Dog("Buddy", 5);
Dog* dog3 = new Dog("Charlie", 1);
// Call member functions on the Dog objects
dog1->bark(); // prints "Woof!"
dog2->setAge(6);
std::cout << dog3->getName() << " is " << dog3->getAge() << " years old." << std::endl; // prints "Charlie is 1 years old."
return 0;
}
// Output:
/*
Woof!
Charlie is 1 years old.
*/
20. C++ Files
In C++, you can use file input/output (I/O) to read and write data to files on your computer. This is useful for storing data that you want to use later, or for reading in data from external sources.
You can open, read, write to the files
#include <fstream>
#include <iostream>
int main() {
// Open a file for reading
std::fstream file;
file.open("data.txt", std::ios::in);
if (!file.is_open()) {
std::cerr << "Error opening file." << std::endl;
return 1;
}
// Read data from the file using the >> operator
int x;
double y;
std::string z;
file >> x >> y >> z;
std::cout << "x: " << x << ", y: " << y << ", z: " << z << std::endl;
// Read a line of text from the file using getline
std::string line;
std::getline(file, line);
std::cout<< "Line: " << line << std::endl;
// Close the file
file.close();
// Open a file for writing
file.open("output.txt", std::ios::out);
if (!file.is_open()) {
std::cerr << "Error opening file." << std::endl;
return 1;
}
// Write data to the file using the << operator
file << x << " " << y << " " << z std::endl;
// Write a block of data to the file using write
char data[10] = {'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j'};
file.write(data, 10);
// Close the file
file.close();
return 0;
}
This program opens a file for reading, reads in data from the file using the >> operator and getline, and then closes the file. It then opens a new file for writing, writes data to the file
21. C++ Exceptions
In C++, exceptions are a mechanism for handling runtime errors that allows you to separate the error handling code from the normal flow of your program. Exceptions can be thrown when an error occurs, and they can be caught and handled by a separate block of code.
To throw an exception in C++, you can use the 'throw' keyword followed by an object or value that represents the exception.
if (x == 0) {
throw std::runtime_error("Division by zero");
}
This code throws a std::runtime_error exception with the message "Division by zero" if x is equal to 0.
To catch an exception in C++, you can use a 'try' block followed by one or more 'catch' blocks. The 'try' block contains the code that might throw an exception, and the 'catch' blocks contain the code that handles the exception.
try {
// this code might throw an exception
int result = 1 / x;
std::cout << result << std::endl;
} catch (std::exception& e) {
// this code is to handle the exception
std::cerr << "Error: " << e.what() << std::endl;
}
This code divides 1 by x and prints the result, but if an exception is thrown, it is caught and an error message is printed to the console.
#include <iostream>
#include <stdexcept>
int divide(int x, int y) {
if (y == 0) {
throw std::runtime_error("Division by zero");
}
return x / y;
}
int main() {
try {
std::cout << divide(10, 2) << std::endl; // prints 5
std::cout << divide(10, 0) << std::endl; // throws an exception
} catch (std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
// Output
/*
5
Error: Division by zero
*/
Thank You
Thank you for visiting and reading through this C++ tutorial. I hope you found it helpful and that you feel more confident working with the language.
If you enjoyed this tutorial, please consider sharing it with others who may also find it useful. Sharing knowledge and helping others learn is a great way to give back to the community.
We have many more tutorials on a variety of programming languages, so please feel free to come back and learn more. We are always happy to help you on your journey to becoming a better programmer.
Thank you again for your time and interest, and I hope you have a great day!