C# (C Sharp) Tutorial
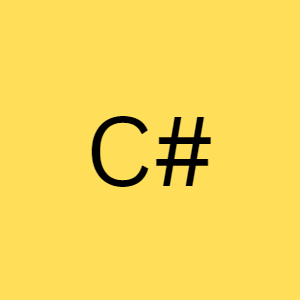
Begin your programming journey with our tutorial The Building Blocks of Computer Programming Designed for beginners, it covers all the essential concepts to help you learn by doing
Concepts:
1. C# Introduction
C# is a modern, object-oriented programming language developed by Microsoft as part of the .NET framework. It is a high-level, statically-typed language that is designed to be easy to read and write, and it is used to build a wide variety of applications, including Windows desktop applications, mobile apps, and web applications.
How to write code for c# ?
To set up a C# development environment, you will need to install the following:
1. A text editor or integrated development environment (IDE) such as Visual Studio, Visual Studio Code, or MonoDevelop.
2. The .NET Framework or .NET Core runtime, which provides a library of classes and functions that you can use in your C# code.
3. The C# compiler, which translates your C# code into machine code that can be executed on a computer.
Once you have these tools installed, you can create a new C# project in your text editor or IDE and start writing C# code.
2. C# Syntax
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!"); // Hello, World!
}
}
}
1. 'using': This directive tells the compiler to include the specified namespace (in this case, 'System') in the program. The 'System' namespace contains many useful classes and functions, including the 'Console' class that we use to output text to the console.
2. 'namespace': A namespace is a container for a set of related classes and functions. In this example, we are defining a namespace called 'HelloWorld' that contains a single class called 'Program'.
3. 'class': A class is a template for an object. In this example, we are defining a class called 'Program' that contains a single function called 'Main'.
4. 'static void Main(string[] args)': This is the entry point for the C# program.
5. 'Console.WriteLine("Hello, World!")': This simple line of code will prints the string "Hello, World!" to the console.
3. C# Output
To output text in C#, you can use the 'Console.Write' or 'Console.WriteLine'
Console.WriteLine("C# webdevmonk tutorial"); // C# webdevmonk tutorial
The above C# program, will print the text "C# webdevmonk tutorial" to the console.
4. C# Comments
In C#, you can use comments to add explanations or notes to your code.
here are two types of comments: single-line comments and multi-line comments.
Single-line comments start with two forward slashes (//) and continue to the end of the line.
int a = 10; // Declare and initialize x
In C# the multi-line comments are begin with a forward slash and an asterisk (/*) and end with an asterisk and a forward slash (*/).
/* This is a
multi-line comment */
You can also use multi-line comments to comment out a block of code.
/*
int a = 10;
int b = 20;
int sum = a + b;
*/
5. C# Variables
In C#, a variable is a storage location for a value of a particular type. You can use variables to store values such as numbers, strings, and objects.
<type> <variableName>;
int a; // declares an integer variable called a
int a = 10; //initialize the variable when you declare it by assigning a value to it using the assignment operator (=)
In C#, you can also declare multiple variables of the same type in a single statement by separating them with commas
int num1, num2, num3;
int num1 = 10, num2 = 20, num3 = 30;
int a; // declares an integer variable
double b; // declares a double-precision floating-point variable
string name; // declares a string variable
bool flag; // declares a Boolean variable
object obj; // declares an object variable
using System;
namespace VariableExample
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize variables
int a = 10;
double b = 20.5;
string name = "John";
bool flag = true;
object obj = new object();
// Print variable values
Console.WriteLine("a = " + b);
Console.WriteLine("b = " +b);
Console.WriteLine("name = " + name);
Console.WriteLine("flag = " + flag);
Console.WriteLine("obj = " + obj);
// Modify variable values
a = 15;
b = 25.5;
name = "Jane";
flag = false;
obj = "Hello, World!";
// Print modified variable values
Console.WriteLine("a = " + a);
Console.WriteLine("b = " + b);
Console.WriteLine("name = " + name);
Console.WriteLine("flag = " + flag);
Console.WriteLine("obj = " + obj);
}
}
}
// Output
/*
a = 10
b = 20.5
name = John
flag = True
obj = System.Object
a = 15
b = 25.5
name = Jane
flag = False
obj = Hello, World!
*/
6. C# Data Types
A data type is a classification of types of data that determine the possible values for that type, the operations that can be performed on it, and how it can be stored in memory.
In C#, there are several built-in datatypes that you can use to declare variables and constants.
bool : A boolean value that can be either true or false.
char : A single Unicode character.
byte : An 8-bit unsigned integer.
sbyte : An 8-bit signed integer.
short : A 16-bit signed integer.
ushort : A 16-bit unsigned integer.
int : A 32-bit signed integer.
uint : A 32-bit unsigned integer.
long : A 64-bit signed integer.
ulong : A 64-bit unsigned integer.
float : A 32-bit single-precision floating point number.
double : A 64-bit double-precision floating point number.
decimal : A 128-bit decimal value.
string : A sequence of Unicode characters.
int num = 5; // Integer (whole number)
double num1 = 5.99D; // Floating point number
char character = 'D'; // Character
bool isTrue = true; // Boolean
string text = "Hello"; // String
using System;
namespace DataTypesExample
{
class Program
{
static void Main(string[] args)
{
// Declare and initialize variables of different data types
int intValue = 10;
double doubleValue = 3.14;
char charValue = 'A';
bool boolValue = true;
string stringValue = "Hello, World!";
Console.WriteLine("intValue: " + intValue);
Console.WriteLine("doubleValue: " + doubleValue);
Console.WriteLine("charValue: " + charValue);
Console.WriteLine("boolValue: " + boolValue);
Console.WriteLine("stringValue: " + stringValue);
}
}
}
// Output:
/*
intValue: 10
doubleValue: 3.14
charValue: A
boolValue: True
stringValue: Hello, World!
*/
7. C# Type Casting
In C#, type casting is the process of converting a value of one data type to another data type. Generally, this can be actually done in either implicitly or explicitly.
Implicit type casting occurs automatically when the value of a variable is assigned to a variable of a different, but compatible, data type.
int a = 10;
double y = a; // Implicit type casting from int to double
In this example, the value of 'a', which is an 'int', is assigned to 'y', which is a 'double'. This is allowed because the 'double' data type is able to hold all the values that the 'int' data type can hold, and therefore the conversion is safe.
Explicit type casting, on the other hand, requires the programmer to specify the data type to which the value should be converted. This is done using parentheses and the name of the target data type.
double a = 3.14;
int y = (int) a; // Explicit type casting from double to int
In this example, the value of 'a', which is a 'double', is explicitly cast to an 'int' and assigned to 'y'. This type of conversion is not always safe, as it may result in data loss if the value of 'a' is not able to be accurately represented as an 'int'.
8. C# Operators
In C#, operators are symbols that perform specific operations on one or more operands.
1. Arithmetic operators
These operators perform arithmetic operations on numerical values, such as addition, subtraction, multiplication, and division.
+, -, *, /, %, ++(increment), --(decrement),
int a = 10;
int b = 20;
int c = a + b; // c is 30
2. Comparison operators:
These operators compare two values and return a Boolean result indicating whether the comparison is true or false.
>, <, >=, <=, ==, !=
int x = 10;
int y = 20;
bool result = x < y; // result is true
3. Logical Operators
These operators perform logical operations on Boolean values, such as AND, OR, and NOT.
bool x = true;
bool y = false;
bool result = x && y; // result is false
4. Assignment Operators
These operators assign a value to a variable.
=, +=(a += 9 same as a = a + 9), -=, *=, /=, %=, &=, |=, ^=, >>=,<<=
int x = 10;
int y = 20;
x = y; // x is now 20
5. Bitwise Operators
These operators perform bitwise operations on integral values, such as AND (&), OR (|), XOR (^), and NOT (!), etc.
int x = 10; // binary representation: 1010
int y = 20; // binary representation: 10100
int z = x & y; // z is 8 (binary representation: 1000)
Example:
using System;
namespace OperatorExample
{
class Program
{
static void Main(string[] args)
{
// Arithmetic operators
int a = 10;
int b = 20;
int c = a + b;
Console.WriteLine("a + b = " + c);
// Comparison operators
bool result = a < b;
Console.WriteLine("a < b is " + result);
// Logical operators
result = a > 0 && b < 30;
Console.WriteLine("a > 0 && y < 30 is " + result);
// Bitwise operators
int a = 10; // binary representation: 1010
int b = 20; // binary representation: 10100
int c = a & b; // c is 8 (binary representation: 1000)
Console.WriteLine("a & b = " + c);
// Assignment operators
a = b;
Console.WriteLine("a = " + a);
}
}
}
// Output
/*
a + b = 30
a < b is True
a > 0 && b < 30 is True
a & b = 8
a = 20
*/
9. C# if, if-else, switch
In C#, you can use the 'if' statement to run a block of code only if a certain condition is true.
int a = 10;
if (a > 5)
{
Console.WriteLine("a is greater than 5"); // a is greater than 5
}
In this example, the code inside the if block will be executed because the condition a > 5 is true.
You can use the if-else statement to execute a block of code if a certain condition is true, and a different block of code if the condition is false.
int a = 10;
if (a > 5)
{
Console.WriteLine("a is greater than 5"); // a is greater than 5
}
else
{
Console.WriteLine("a is not greater than 5");
}
In this example, the code inside the first if block will be executed because the condition a > 5 is true.
You can also use the switch statement to execute a block of code based on the value of a variable. The switch statement allows you to specify multiple cases, each with its own block of code to execute.
int a = 2;
switch (a)
{
case 1:
Console.WriteLine("a is 1");
break;
case 2:
Console.WriteLine("a is 2"); // a is 2
break;
case 3:
Console.WriteLine("a is 3");
break;
default:
Console.WriteLine("a is not 1, 2, or 3");
break;
}
In the above example program, the code inside the case 2: block will be executed because the value of a is 2.
10. C# while, do-while, for loops
while loop
A 'while' loop repeatedly executes a block of code as long as a certain condition is true.
//Syntax
while (condition)
{
// code block to execute
}
int i = 0;
while (i < 10){
Console.WriteLine(i);
i++;
}
This will print the numbers 0 through 9 to the console.
do-while loop
A do-while loop is similar to a while loop, but the code block will always execute at least once before the condition is checked.
// Syntax
do
{
// code block to execute
} while (condition);
int i = 0;
do
{
Console.WriteLine(i);
i++;
} while (i < 10);
This will also print the numbers 0 through 9 to the console.
int i = 1;
do
{
Console.WriteLine("I can print even though the while condition is false");
i++;
} while (i > 10);
The body of the loop will execute even though the while condition is false because do-while loop execute first before checking the condition.
for loop
A for loop allows you to specify a loop counter and the conditions for terminating the loop.
for (initialization; condition; iteration)
{
// code block to execute
}
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i);
}
/*
0
1
2
3
4
5
6
7
8
9
*/
This will also print the numbers 0 through 9 to the console.
foreach loop
You can also use the 'foreach' loop to iterate over a collection or array in C#.
foreach (var item in collection)
{
// code block to execute
}
int[] numbers = { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
This will print the numbers from 1 to 5 to the console.
11. C# break and continue
break
The 'break' statement is used to exit a loop early at a specific condition, before the loop condition is 'false'. When the 'break' statement is encountered inside a loop, the loop is immediately terminated and the program continues with the next statement after the loop.
continue
The 'continue' statement is used to skip the rest of the current iteration of a loop and move on to the next iteration. When the 'continue' statement is encountered inside a loop, the program jumps to the next iteration of the loop, without executing the remaining statements in the current iteration.
for (int i = 0; i < 10; i++)
{
if (i % 2 == 0)
{
continue; // skip the rest of the current iteration
}
if (i == 7)
{
break; // exit the loop
}
Console.WriteLine(i);
}
/*
Output:
13
5
*/
12. C# Booleans
In C#, a boolean is a type that can represent one of two values: 'true' or 'false'. Booleans are often used in conditional statements to test a condition and execute different code blocks depending on the result of the test.
bool flag = true;
if (flag)
{
Console.WriteLine("flag is true"); // flag is true
}
else
{
Console.WriteLine("flag is false");
}
Remember, 'if' statement will print it's block only if the condition is true other 'else' block of code will execute.
This will print "flag is true" to the console.
You can also use boolean values in expressions, such as:
bool a = true;
bool b = false;
bool c = a && b; // c is false
bool d = a || b; // d is true
The && operator represents a logical AND, which is true if both operands are true, and false otherwise.
int a = 5;
int b = 10;
bool c = a < b; // c is true
In this example, 'c' is true because 'a' is less than 'b'.
13. C# Arrays
In C#, an array is a collection of elements of the same type, stored in a contiguous block of memory. You can declare an array by specifying the type of its elements and the number of elements in square brackets
int[] numbers = new int[5];
This creates an array of ints with 5 elements, which are initialized to the default value for their type (0 for integers).
You can also initialize an array with a list of values
int[] numbers = new int[] { 1, 2, 3, 4, 5 };
Or you can use like this
int[] numbers = { 1, 2, 3, 4, 5 };
Once you have an array, you can access its elements using an index in square brackets,
int first = numbers[0]; // first is 1
int last = numbers[4]; // last is 5
Note that array indices are zero-based, so the first element of an array is at index 0, the second element is at index 1, and so on.
You can also use a loop to iterate over the elements of an array
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
This will print the numbers 1 through 5 to the console.
You can also use the 'foreach' loop to iterate over an array:
foreach (int number in numbers)
{
Console.WriteLine(number);
}
This will also print the numbers 1 through 5 to the console.
using System;
namespace ArrayExample
{
class Program
{
static void Main(string[] args)
{
// create an array of strings
string[] names = { "Alice", "Bob", "Charlie", "Dave", "Eve" };
// print the elements of the array
foreach (string name in names)
{
Console.WriteLine(name);
}
// to modify an element of array
names[3] = "Carol";
// print the modified array
Console.WriteLine("Modified array:");
foreach (string name in names)
{
Console.WriteLine(name);
}
}
}
}
// Output
/*
Alice
Bob
Charlie
Dave
Eve
Modified array:
Alice
Bob
Charlie
Carol
Eve
*/
14. C# Strings
In C#, the 'string' is a sequence of characters. It is a reference type, which means that a variable of type "string" stores a reference to the location of the string in memory, rather than the string itself. This means that strings are mutable, which means that you can change the value of a string after it is created.
// Creating a string
string str1 = "Hello, World!";
// Concatenating strings
string str2 = "Hello, ";
string str3 = "World!";
string str4 = str2 + str3; // str4 is "Hello, World!"
// Accessing characters in a string
char firstChar = str1[0]; // firstChar is 'H'
char lastChar = str1[str1.Length - 1]; // lastChar is '!'
// Getting a substring
string str5 = str1.Substring(7, 5); // str5 is "World"
// Comparing strings (case-sensitive)
bool areEqual = str1 == str4; // areEqual is true
// Comparing strings (case-insensitive)
bool areEqualIgnoreCase = str1.Equals(str4, StringComparison.OrdinalIgnoreCase); // areEqualIgnoreCase is true
// Checking if a string starts with or ends with a specific prefix or suffix
bool startsWithH = str1.StartsWith("H"); // startsWithH is true
bool endsWithExclamation = str1.EndsWith("!"); // endsWithExclamation is true
C# also provides the StringBuilder class, which allows you to efficiently build up a string by appending new characters or substrings to it.
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10; i++) {
sb.Append("Hello, World! ");
}
string str = sb.ToString(); // str is "Hello, World! Hello, World! Hello, World! Hello, World! Hello, World! Hello, World! Hello, World! Hello, World! Hello, World! Hello, World! "
15. C# Methods
In C#, a method is a block of code that can perform a specific task and may return a result when it is called. Methods are declared within a class or struct and can be called (invoked) from other parts of the program.
public class Calculator {
public int Add(int a, int b) {
return a + b;
}
}
This example declares a class called 'Calculator' with a method called 'Add' that takes two int arguments and returns the sum of the two numbers as an integer result.
To call (invoke) a method, you can use the method name followed by the arguments in parentheses.
Calculator calculator = new Calculator();
int result = calculator.Add(3, 4); // result is 7
Methods can also have optional parameters, which allow you to omit certain arguments when calling the method. Optional parameters must be assigned a default value in the method declaration.
public int Multiply(int x, int y, int z = 1) {
return a * b * c;
}
int result1 = calculator.Multiply(2, 3); // result1 is 6 (c is 1 by default)
int result2 = calculator.Multiply(2, 3, 4); // result2 is 24 (c is 4)
Complete example:
using System;
namespace MethodExample
{
public class Calculator
{
// Method that adds two numbers and returns the result
public int Add(int a, int b)
{
return a + b;
}
// Method that subtracts two numbers and returns the result
public int Subtract(int x, int y)
{
return a - b;
}
// Method that multiplies two numbers and returns the result
// with an optional third parameter that defaults to 1
public int Multiply(int x, int y, int z = 1)
{
return a * b * c;
}
// Method that divides two numbers and returns the quotient and remainder
// using output parameters
public void Divide(int numerator, int denominator, out int quotient, out int remainder)
{
quotient = numerator / denominator;
remainder = numerator % denominator;
}
}
class Program
{
static void Main(string[] args)
{
Calculator calculator = new Calculator();
int result1 = calculator.Add(3, 4);
Console.WriteLine("3 + 4 = " + result1);
int result2 = calculator.Subtract(10, 5);
Console.WriteLine("10 - 5 = " + result2);
int result3 = calculator.Multiply(2, 3);
Console.WriteLine("2 * 3 = " + result3);
int result4 = calculator.Multiply(2, 3, 4);
Console.WriteLine("2 * 3 * 4 = " + result4);
int quotient;
int remainder;
calculator.Divide(10, 3, out quotient, out remainder);
Console.WriteLine("10 / 3 = " + quotient + " remainder " + remainder);
}
}
}
// Output:
/*
3 + 4 = 7
10 - 5 = 5
2 * 3 = 62 * 3 * 4 = 24
10 / 3 = 3 remainder 1
*/
16. C# OOP Concepts
Object-oriented programming (OOP) is a programming paradigm that is based on the concept of "objects", which are data structures that contain both data and behavior.
1. Classes
A 'class' is a template or blueprint for creating many objects. It defines the data and behavior that objects of that class will have.
public class Employee
{
public string Name { get; set; }
public int Age { get; set; }
public string Department { get; set; }
public void Work()
{
Console.WriteLine("Doing some work...");
}
}
2. Object
An object is an instance of a class. You can create an object by using the 'new' keyword and calling the class's constructor.
Employee employee = new Employee();
employee.Name = "John Smith";
employee.Age = 30;
employee.Department = "IT";
employee.Work();
3. Constructors
A constructor is a special method that is called only when the object is created. Constructors are used to initialize the data of an object and set it up for use.
There are two main types of constructors in C#: parameterized constructors and default constructors.
public class Employee
{
public string Name { get; set; }
public int Age { get; set; }
public string Department { get; set; }
public Employee(string name, int age, string department)
{
Name = name;
Age = age;
Department = department;
}
}
Employee employee = new Employee("John Smith", 30, "IT");
4. Inheritance
Inheritance is a special model to create a new class that is a updated version of an existing class. The new class is called the derived class or child class, and the existing class is the base class or parent class. The derived class can inherit the data and behavior of the base class and can also have additional data and behavior of its own.
public class Manager : Employee
{
public int NumberOfEmployees { get; set; }
public void Lead()
{
Console.WriteLine("Leading my team...");
}
}
5. Polymorphism
Polymorphism is the ability of a class to be used in multiple forms. There are two main types of polymorphism in C#: inheritance-based polymorphism (also known as "overriding") and interface-based polymorphism (also known as "implementing").
6. Encapsulation
Encapsulation is the concept of bundling data and behavior together in a single unit, or object.
public class Employee
{
private string name;
private int age;
private string department;
public Employee(string name, int age, string department)
{
this.name = name;
this.age = age;
this.department = department;
}
public string Name
{
get { return name; }
set { name = value; }
}
public int Age
{
get { return age; }
set { age = value; }
}
public string Department
{
get { return department; }
set { department = value; }
}
public void Work()
{
Console.WriteLine(Name + " is doing some work in the " + Department + " department.");
}
}
example:
using System;
namespace ClassExample
{
public class Employee
{
public string Name { get; set; }
public int Age { get; set; }
public string Department { get; set; }
public Employee(string name, int age, string department)
{
Name = name;
Age = age;
Department = department;
}
public void Work()
{
Console.WriteLine(Name + " is doing some work in the " + Department + " department.");
}
}
class Program
{
static void Main(string[] args)
{
Employee employee1 = new Employee("John Smith", 30, "IT");
Employee employee2 = new Employee("Jane Doe", 35, "Marketing");
employee1.Work();
employee2.Work();
}
}
}
// Output
/*
John Smith is doing some work in the IT department.
Jane Doe is doing some work in the Marketing department.
*/
17. C# Files
In C#, a file is a named location on a disk that stores data, programs, or other information. C# provides a set of classes in the 'System.IO' namespace that you can use to read, write, and manipulate files in your code.
Here is an example of how to read a text file in C# using the 'StreamReader' class:
using System;
using System.IO;
namespace FileExample
{
class Program
{
static void Main(string[] args)
{
string filePath = "C:\\temp\\myfile.txt";
string fileContent;
using (StreamReader reader = new StreamReader(filePath))
{
fileContent = reader.ReadToEnd();
}
Console.WriteLine(fileContent);
}
}
}
Here is an example of how to write to a text file in C# using the 'StreamWriter' class:
using System;
using System.IO;
namespace FileExample
{
class Program
{
static void Main(string[] args)
{
string filePath = "C:\\temp\\myfile.txt";
string fileContent = "Hello, World!";
using (StreamWriter writer = new StreamWriter(filePath))
{
writer.Write(fileContent);
}
}
}
}
There are many other classes and methods in the System.IO namespace that you can use to work with files in C#.
18. C# enum
In C#, an 'enum' (enumeration) is a value type that represents a set of named constants. Enums are useful for defining a set of related values that can be used throughout your code, and can help to make your code more readable and maintainable by providing named constants instead of magic numbers.
using System;
namespace EnumExample
{
public enum DaysOfTheWeek
{
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday,
Sunday
}
class Program
{
static void Main(string[] args)
{
DaysOfTheWeek today = DaysOfTheWeek.Monday;
if (today == DaysOfTheWeek.Saturday || today == DaysOfTheWeek.Sunday)
{
Console.WriteLine("It's the weekend!");
}
else
{
Console.WriteLine("It's a weekday.");
}
}
}
}
In this example, an 'enum' called DaysOfTheWeek is defined with seven named constants representing the days of the week. The enum is then used to determine whether a given day is a weekday or a weekend day.
19. C# Input
In C#, you can use the 'Console' class in the 'System' namespace to read input from the console. The 'Console' class provides several methods for reading input, depending on the type of data you want to read.
using System;
namespace InputExample
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter your name: ");
string name = Console.ReadLine();
Console.WriteLine("Hello, " + name + "!");
}
}
}
This example uses the ReadLine method of the 'Console' class to read a string from the console. The 'ReadLine' method reads a line of text from the console and returns it as a string.
Here is an example of how to read a number from the console in C#
using System;
namespace InputExample
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter a number: ");
int number = int.Parse(Console.ReadLine());
Console.WriteLine("You entered: " + number);
}
}
}
This example uses the 'ReadLine' method to read a string from the console, and then uses the 'int.Parse' method to convert the string to an integer.
You can also use the ReadKey method to read a single key from the console without blocking the program.
using System;
namespace InputExample
{
class Program
{
static void Main(string[] args)
{
ConsoleKeyInfo key = Console.ReadKey();
Console.WriteLine("You pressed: " + key.KeyChar);
}
}
}
This example uses the 'ReadKey' method to read a single key from the console and stores it in a 'ConsoleKeyInfo' object. The KeyChar property of the 'consoleKeyInfo' object contains the character representation of the key that was pressed.
Thank You
Thank you for taking the time to go through the webdevmonk's 45 minute C# tutorial. We hope that you found the tutorial helpful and informative. If you enjoyed this tutorial, we encourage you to check out some of our other tutorials on our website. We have a variety of topics covered, and we're sure you'll find something else that interests you.
In addition to our tutorials, don't forget to visit our project page for access to free source code. This can be a great way to practice what you've learned and to see how it can be applied in a real-world setting.
Finally, we recommend re-reading this tutorial to fully understand the concepts covered. Practice makes perfect, and the more you go over the material, the better you'll understand it.
Thank you again for your interest in our tutorials. We hope to see you again soon!