Dart Tutorial
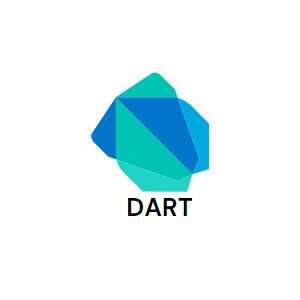
Begin your programming journey with our tutorial The Building Blocks of Computer Programming Designed for beginners, to help you learn by doing
Concepts:
1. Dart Introduction
Dart is a programming language developed by Google. It is a general-purpose language that is used to build applications for the web, mobile, and desktop.
One of the main advantages of Dart is its ability to run on multiple platforms. With the use of Flutter, a mobile app development framework created by Google, it is possible to build natively compiled applications for mobile, web, and desktop from a single codebase. This means that developers can write their code once and deploy it to different platforms, which can save time and resources.
Dart is also designed to be easy to learn and use. It has a syntax that is similar to other popular programming languages such as C# and Java, which makes it accessible to a wide range of developers. It also has strong support for object-oriented programming and includes features such as interfaces, abstract classes, and mixins.
Dart is used in a number of Google products, including the Flutter framework for building mobile apps and the Angular framework for building web applications. It is also used by other companies and organizations for building a variety of applications.
Careers:
1. 'Mobile app developer': Dart is the primary programming language used in the Flutter framework, which is used to build natively compiled mobile apps for Android and iOS.
2. 'Full-stack developer': Some developers may choose to use Dart as their language of choice for both front-end and back-end development. Full-stack developers with expertise in Dart may be able to work on a wide range of projects, from mobile apps to web applications and beyond.
3. 'Data scientist': Dart has strong support for machine learning and data analysis, and developers with expertise in these areas may be able to use Dart to build applications that process and analyze large amounts of data.
Environment setup for Dart
To set up a development environment for Dart, you will need to install the Dart SDK (Software Development Kit) on your computer. The Dart SDK includes the Dart runtime and command-line tools that you can use to develop, run, and test Dart applications
Download the latest stable release of the Dart SDK from the official website //dart.dev/get-dart.
Once you installed Dart on your machine, then you can start write Code in Dart
To write code in Dart, you can use any text editor or integrated development environment (IDE) that supports Dart. Some popular IDEs for Dart development include Visual Studio Code, Android Studio, and WebStorm.
Here are some basic steps to write and run a simple Dart program
1. Open your favourite text editor or any IDE and create a new dart file.
2. Write the following Dart code in the file
void main() {
print('Hello, World!');
}
3. Save the file as 'main.dart'.
4. Open a terminal window and navigate to the directory where you saved the file
5. Run the following command to run the Dart program
dart main.dart
This will execute the main function, which will print "Hello, World!" to the console.
2. Dart Syntax
Dart has a C-style syntax, which means that it uses curly braces to enclose code blocks and semicolons to terminate statements. It also supports object-oriented programming with classes and interfaces, and includes features such as generics, mixins, and asynchronous programming with async/await.
Here is a simple example of a Dart program that prints "Dart Tutorial by webdevmonk" to the console
void main() {
print('Dart Tutorial by webdevmonk');
}
In Dart, the 'main' function is the entry point of the program. The 'void' keyword in the above Dart program tells that the function does not return a value. The print function is used to output a string to the console.
Dart also supports top-level functions, variables, and other declarations outside of class definitions. For example, the following code defines a top-level variable and a function that returns the sum of two numbers
int number = 5;
int add(int a, int b) {
return a + b;
}
void main() {
print(add(number, 3)); // prints 8
}
3. Dart Comments
In Dart, you can use the comments to describe and notes to your code. Comments are ignored by the Dart compiler and do not affect the behavior of the program.
There are two types of comments in Dart: single-line comments and multi-line comments.
// This is a single-line comment
/*
This is a
multi-line comment
*/
Here is an example of using comments in a Dart program
void main() {
// Print the current time
print(DateTime.now());
/*
Calculate the sum of two numbers.
value stored in the 'sum' variable.
*/
int sum = 1 + 2;
print(sum); // prints 3
}
4. Dart Ouput
You might already familiar with this.
In Dart, you can use the 'print' function to output strings, numbers, and other values to the console. The 'print' function takes a single argument and prints it to the console, followed by a newline character.
void main() {
print('Hello, World!'); // output a string
print(42); // output a number
print(true); // output a boolean
print([1, 2, 3]); // output a list
}
// Output:
/*
Hello, World!
42
true
[1, 2, 3]
*/
If you want to print multiple values on the same line, you can use string interpolation to embed the values in a string.
void main() {
int a = 1;
int b = 2;
print('The sum of $a and $b is ${a + b}'); // output 'The sum of 1 and 2 is 3'
}
Here, the $a and $b expressions are replaced with the values of the a and b variables, and the ${a + b} expression is replaced with the result of the a + b expression.
5. Dart Variables
In Dart, variables are used to store the values in a computer memory. A variable has a name and a type, and it can hold a value of that type.
To declare a variable in Dart, you use the var keyword followed by the variable name
var name = 'John'; // name is a string variable
var age = 30; // age is an int variable
var isEmployed = true; // isEmployed is a boolean variable
You can also specify the type of a variable explicitly using the 'Type' syntax.
String name = 'John'; // name is a string variable
int age = 30; // age is an int variable
bool isEmployed = true; // isEmployed is a boolean variable
If you declare a variable but do not assign a value to it, the variable is initialized to the default value for its type.
int a; // a is initialized to 0
bool b; // b is initialized to false
You can reassign a new value to a variable using the assignment operator (=)
var name = 'John';
name = 'Jane'; // name is now 'Jane'
You can also declare multiple variables of the same type in a single statement using the 'Type' syntax.
int a, b, c; // a, b, and c are all int variables
Rules for declaring variable in Dart
These are some the rules to declare a variable in Dart
1. Variable names can contain letters, digits, and underscores (_). They must start with a letter or an underscore.
2. Variable names in Dart are case-sensitive. For example, name and Name are different variables.
3. Variable names cannot be the same as a reserved keyword in Dart. For example, you cannot use 'var' or 'if' as a variable name.
4. Variable names should follow the naming conventions of the Dart style guide. In general, variables should be written in lowerCamelCase, with the first letter of each word except the first one being capitalized.
// valid variable names
var name;
var _private;
var userId;
// invalid variable names
var 123; // must start with a letter or underscore
var if; // cannot be a reserved keyword
var user-id; // cannot contain hyphens
6. Dart Built-in-types
Dart has several built-in types that represent different kinds of values, such as numbers, strings, and booleans.
Numbers
Dart has two types for representing numbers: 'int' (for integers) and 'double' (for floating-point numbers)
int x = 42; // x is an int with the value 42
double y = 3.14; // y is a double with the value 3.14
Strings
Strings are sequences of Unicode characters. You can use single quotes (') or double quotes (") to define a string.
String s1 = 'Hello, World!';
String s2 = "Hello, World!";
Booleans
Booleans represent true or false values. The bool type has only two values in dart: 'true' and 'false'.
bool isTrue = true;
bool isFalse = false;
Lists
Lists (also known as arrays) are ordered collections of values. You can define a list using the List type and enclosing the values in square brackets ([]).
List<int> numbers = [1, 2, 3]; // numbers is a list of integers
List<String> names = ['John', 'Jane', 'Bob']; // names is a list of strings
Sets
Sets are unordered collections of unique values. You can define a set using the Set type and enclosing the values in curly braces ({}).
Set<int> numbers = {1, 2, 3}; // numbers is a set of integers
Set<String> names = {'John', 'Jane', 'Bob'}; // names is a set of strings
Maps
Maps are collections of key-value pairs. You can define a map using the Map type and enclosing the key-value pairs in curly braces ({}).
Map<String, int> grades = {'John': 80, 'Jane': 90, 'Bob': 70}; // grades is a map of strings to integers
Map<int, String> names = {1: 'John', 2: 'Jane', 3: 'Bob'}; // names is a map of integers to strings
Runes and grapheme Clusters
In Dart, 'runes' are a way to represent Unicode characters using integers. Runes are useful when you need to manipulate individual Unicode characters, such as when working with internationalization or text processing.
Grapheme clusters are sequences of Unicode characters that are treated as a single unit by a user's operating system. For example, the character "é" (U+00E9) is composed of two Unicode code points: "e" (U+0065) and "´" (U+0301). When displayed on a screen, the two code points are combined to form a single glyph that represents the character "é".
In Dart, you can use the 'String.split' function to split a string into a list of grapheme clusters.
void main() {
var string = 'Hello, World!';
var graphemeClusters = string.split(''); // graphemeClusters is a list of strings, each representing a grapheme cluster
print(graphemeClusters); // prints ['H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!']
}
Symbols
In Dart, symbols are unique, immutable objects that represent a specific instance of a name. Symbols are often used to identify a name in a more efficient and reliable way than using a string.
To create a symbol in Dart, you can use the '#' operator followed by the name.
var symbol1 = #hello; // symbol1 is a symbol representing the name 'hello'
var symbol2 = #world; // symbol2 is a symbol representing the name 'world'
You can also create a symbol from a string using the 'Symbol' class
var symbol = Symbol('hello'); // symbol is a symbol representing the name 'hello'
Symbols are often used as keys in maps and as identifiers in mirrors (a feature of the Dart runtime for reflection and introspection).
7. Dart Operators
In Dart, the operators are special symbols that can perform operations on one or more operands (variables or values). Operators can be used to perform arithmetic calculations, assign values to variables, compare values, and more.
Arithmetic Operators
These operators perform arithmetic calculations on numerical operands. They include + (addition), - (subtraction), * (multiplication), / (division), ~/ (integer division), % (modulo division)
var a = 1 + 2; // a is 3
var b = 5 - 3; // b is 2
var c = 2 * 3; // c is 6
var a = 10 / 3; // a is 3.3333333333333335
var b = 10 ~/ 3; // b is 3 (integer division)
var c = 10 % 3; // c is 1 (remainder of the division)
var d = -a; // d is -3
Assignment operators
These operators assign a value to a variable. They include = (assignment), ??= (null-aware assignment), and compound assignment operators such as +=, -=, *=, and /=.
var a = 1; // a is 1
a = 2; // a is now 2
var b; // b is null
b ??= 3; // b is now 3
var c = 1;
c += 2; // c is now 3
Comparison operators
These operators compare two values and return a boolean result. They include == (equal to), != (not equal to), > (greater than), >= (greater than or equal to), < (less than), and <= (less than or equal to).
var a = 1;
var b = 2;
print(a == b); // prints false
print(a != b); // prints true
print(a > b); // prints false
print(a >= b); // prints false
print(a < b); // prints true
print(a <= b); // prints true
Logical operators
These operators perform logical operations on boolean operands. They include && (and), || (or), and ! (not).
var a = true;
var b = false;
print(a && b); // prints false
print(a || b); // prints true
print(!(a || b)) // prints false
Type test operators
In Dart, you can use the 'is' and 'is!' operators to test the type of an object.
The 'is' operator returns true if the object is an instance of the specified type, and false otherwise.
var a = 'Hello';
print(a is String); // prints true
print(a is int); // prints false
The is! operator returns true if the object is not an instance of the specified type, and false otherwise.
void printType(dynamic value) {
if (value is String) {
print('The value is a string');
} else if (value is int) {
print('The value is an integer');
} else {
print('The value is of unknown type');
}
}
printType('Hello'); // prints 'The value is a string'
printType(123); // prints 'The value is an integer'
printType(null); // prints 'The value is of unknown type'
Cascade notation
In Dart, the cascade notation (also known as the "cascade operator" or the "cascade syntax") is a way to perform multiple operations on the same object in a single expression
The cascade notation uses the .. operator to chain together a series of method calls or property assignments. The object on which the operations are performed is specified at the beginning of the expression, and the operations are separated by .. operators.
Here is an example of using the cascade notation to chain together a series of method calls
var sb = StringBuffer()
..write('Hello')
..write(' ')
..write('World');
print(sb.toString()); // prints 'Hello World'
In the example above, the 'StringBuffer' object is created at the beginning of the expression, and then three method calls are chained together using the cascade notation.
Conditional Operator
In Dart, the conditional operator is expressed using the question mark "(?)" and colon "(:)" symbols
This operator is also known as the ternary operator, because it takes three operands: the condition, expression1 (which is evaluated if the condition is true), and expression2 (which is evaluated if the condition is false).
int a = 10;
int b = 5;
int result = a > b ? a : b;
print(result); // Output: 10
In this example, the condition a > b evaluates to true, so the expression a is assigned to the variable result. The output of the print statement is 10.
8. Dart Input
In Dart, you can use the stdin object from the 'dart:io' library to read input from the standard input stream (usually the keyboard).
import 'dart:io';
void main() {
stdout.write('Enter your name: '); // prompt the user for input
String name = stdin.readLineSync(); // read a line of input
print('Hello, $name!'); // print the input
}
In the example above, the 'stdout.write' function is used to print a prompt to the user, and the 'stdin.readLineSync' function is used to read a line of input from the user. The input is then stored in the name variable and printed to the console using the 'print' function.
You can also use the stdin.readSync function to read a single character of input from the user
import 'dart:io';
void main() {
stdout.write('Enter a character: '); // prompt the user for input
int character = stdin.readSync(); // read a single character of input
print('You entered the character "${String.fromCharCode(character)}".'); // print the input
}
Note that the 'readLineSync' and 'readSync' functions are synchronous, which means that they block the program until the user enters some input. If you want to read input asynchronously, you can use the 'stdin.readLine' and 'stdin.read' functions, which return a 'Future' object
9. Dart if, if-else, switch
In Dart, you can use the 'if' and 'if-else' statements to control the flow of your program based on a condition
if
The 'if' statement in dart will execute a block of code if the condition is only true.
var a = 10;
if (a > 0) {
print('a is positive');
}
if-else
You can also use the if-else statement to specify an alternative block of code to execute if the condition is not true
var a = -10;
if (a > 0) {
print('a is positive');
} else {
print('a is not positive');
}
In the example above, the if statement checks if the value of x is greater than zero, and if it is, it executes the code inside the first set of curly braces. If the condition is not met, the code inside the second set of curly braces is executed.
switch
You can also use the 'switch' statement to evaluate a value and execute code based on a matching case. The 'switch' statement is often used as an alternative to a series of 'if-else' statements.
var a = 'hello';
switch (a) {
case 'hello':
print('a is "hello"');
break;
case 'world':
print('a is "world"');
break;
default:
print('a is something else');
}
In the example above, the 'switch' statement evaluates the value of x and executes the code inside the matching case block. If no case matches the value of x, the code inside the 'default' case is executed. The break statement is used to exit the switch statement and prevent the code from falling through to the next case.
10. Dart while, do-while, for
while loop
The 'while' loop can executes a block of code multiple times as long as the condition is true.
var count = 0;
while (count < 5) {
print(count);
count++;
}
In the example above, the 'while' loop will execute the code inside the curly braces until the value of count is no longer less than 5. The count++ expression increases the value of count by 1 after each iteration of the loop
do-while loop
The 'do-while' loop is similar to the 'while' loop, but it guarantees that the code inside the loop will be executed at least once, even if the condition is false
var count = 0;
do {
print(count);
count++;
} while (count < 5);
In the example above, the 'do-while' loop will execute the code inside the curly braces at least once, and then it will continue to execute the code as long as the value of 'count' is < 5.
for loop
The 'for' loop in dart, is used to execute a block of code in a specific number of times as the condition is true.
for (var i = 0; i < 5; i++) {
print(i);
}
example for all loops
void main() {
// While loop
var count = 0;
while (count < 5) {
print('while: $count');
count++;
}
// Do-while loop
count = 0;
do {
print('do-while: $count');
count++;
} while (count > 5); // count is not greater than 5, even though the condition is false it will execute once
// For loop
for (var i = 0; i < 5; i++) {
print('for: $i');
}
}
// Output
/*
while: 0
while: 1
while: 2
while: 3
while: 4
do-while: 0
for: 0
for: 1
for: 2
for: 3
for: 4
*/
In the example above, the 'for' loop will execute the code inside the curly braces 5 times, with the value of i starting at 0 and increasing by 1 each time. The loop will continue as long as the value of i is less than 5.
11. Dart break and continue
In Dart, you can use the break and continue statements to control the flow of a loop.
break
The 'break' statement in Dart is used to exit a loop and continue execution of the code after the loop.
Here is an example of using the 'break' statement in a while' loop
var count = 0;
while (true) {
if (count >= 5) {
break;
}
print(count);
count++;
}
print('Done');
In the above Dart example, the 'while' loop will run indefinitely until the break statement is encountered. When the value of count becomes greater than or equal to 5, the break statement is executed, and the loop is terminated. The code after the loop (print('Done')) is then executed.
continue
The 'continue' statement is used to skip the rest of the current iteration of a loop and continue with the next iteration. Here is an example of using the 'continue' statement in a for loop
for (var i = 0; i < 10; i++) {
if (i % 2 == 0) {
continue;
}
print(i);
}
In the example above, the 'for' loop iterates over a range of numbers from 0 to 9. If the value of i is even (that is, if i is divisible by 2), the continue statement is executed, and the rest of the loop's code is skipped. Otherwise, the value of i is printed to the console. The output of the loop will be the odd numbers from 1 to 9.
12. Dart Assert
In Dart, you can use the 'assert' function to verify that a boolean expression is true at runtime. If the expression is false, the 'assert' function will throw an exception.
void main() {
var a = 10;
var b = 20;
assert(a < b); // this assertion will pass
assert(a > b); // this assertion will fail
}
In the example above, the first 'assert' function call will pass because the expression a < b is true. The 'second' assert function call will fail because the expression a > b is false. When the 'assert' function is called with a false expression, it will throw an AssertionError exception
You can also pass a second argument to the 'assert' function to specify a message to be included in the exception
void main() {
var a = 10;
var b = 20;
assert(a > b, 'a should be greater than b'); // this assertion will fail
}
In the example above, when the 'assert' function is called with a false expression, it will throw an AssertionError exception with the message "x should be greater than y".
13. Dart exceptions
In Dart, an exception is an abnormal event that occurs during the execution of a program and disrupts the normal flow of control. Exceptions can be thrown by the Dart runtime, by the standard library, or by your own code.
When an exception is thrown, it can be caught and handled by a 'try-catch' block. The 'try' block contains the code that may throw an exception, and the 'catch' block contains the code that handles the exception
Here is an example of using a 'try-catch' block to handle an exception
void main() {
try {
int.parse('abc'); // this will throw a FormatException
} catch (e) {
print('Caught exception: $e');
}
}
In the example above, the 'try' block contains a call to the 'int.parse' function, which attempts to parse a string as an integer. If the string is not a valid integer, the 'int.parse' function will throw a FormatException. The 'catch' block will catch the exception and print a message to the console.
You can also specify multiple 'catch' blocks to handle different types of exceptions
void main() {
try {
int.parse('abc'); // this will throw a FormatException
} on FormatException catch (e) {
print('Caught FormatException: $e');
} on Exception catch (e) {
print('Caught Exception: $e');
}
}
In the example above, the first 'catch' block will handle FormatExceptions, and the second 'catch' block will handle all other types of exceptions. If an exception is thrown that is not a FormatException, it will be caught by the second 'catch' block
You can also use the 'finally' block in Dart to specify code that should be executed regardless of whether an exception is thrown or caught. The 'finally' block is optional, and it is typically used to clean up resources or perform other tasks that should be done regardless of the outcome of the 'try' block
void main() {
try {
int a = int.parse('hello'); // this will throw a FormatException
} catch (e) {
print('Caught exception: $e');
} finally {
print('Executing finally block');
}
}
In the example above, the 'finally' block will be executed after the 'catch' block, regardless of whether an exception was thrown or caught.
14. Dart Functions
A function is a collection of reusable code that can be execute whenever it is called in the program.
void printHello() {
print('Hello, World!');
}
void main() {
printHello();
printHello();
printHello();
}
// Ouput
/*
Hello, World!
Hello, World!
Hello, World!
*/
In the example above, the printHello function is defined to print the string "Hello, World!" to the console. The function is called using its name followed by a set of parentheses.
You can also define functions with parameters and return values
int add(int a, int b) {
return a + b;
}
void sayHi(String name) {
print("Hi, ${name}");
}
void main() {
int result = add(10, 20);
print(result); // prints 30
sayHi("Martha");
}
// Ouput
/*
30
Hi, Martha
*/
You can also specify default values for function parameters, which allows you to call the function with fewer arguments
int add(int a, int b, [int c = 0]) {
return a + b + c;
}
void main() {
int result1 = add(10, 20); // z is 0
int result2 = add(10, 20, 30); // z is 30
print(result1); // prints 30
print(result2); // prints 60
}
In the Dart programming language, you can use the fat arrow (=>) syntax to define a function or method with a concise body. This is often called the "short-hand function syntax" or "fat arrow function syntax".
Here is an example of how to use the fat arrow syntax to define a function
add(int x, int y) => x + y;
This is equivalent to the following function definition using the 'function' keyword
int add(int a, int b) {
return a + b;
}
You can use the fat arrow syntax to define a function with a single line of code. If the function body is longer, you can use the {} syntax to define a block of code.
doSomething() => print('Hello, World!');
doSomethingElse() {
print('Hello, World!');
print('Goodbye, World!');
}
15. Dart Classes
In the Dart programming language, a class is a template for creating multiple objects. A class can contain properties (variables) and methods (functions) that describe the characteristics and behavior of objects created from the class.
class Student {
String name;
int age;
double gradePointAverage;
void study() {
print('$name is studying');
}
void sleep() {
print('$name is sleeping');
}
}
This class defines a Student type that has three properties: name, age, and gradePointAverage. It also has two methods: study() and sleep().
o create an object (an instance) of the Student class, you can use the new keyword followed by the class name and parentheses
Student student = new Student();
You can then access the object's properties and methods using the dot notation
student.name = 'Alice';
student.age = 20;
student.gradePointAverage = 3.8;
student.study(); // Output: Alice is studying
student.sleep(); // Output: Alice is sleeping
16. Dart Constructors
In the Dart programming language, a constructor is a special method that is called when an object is created from a class. A class can have multiple constructors, which allows you to create objects in different ways.
Here is an example of a class with a single constructor
class Point {
num a, b;
Point(this.a, this.b);
}
To create an object of the Point class, you can call the constructor like this
Point p = Point(1, 2);
You can also define multiple constructors in a class by using the 'factory' keyword.
class Point {
num a, b;
Point(this.a, this.b);
factory Point.fromJson(Map json) {
return Point(json['a'], json['b']);
}
}
In this example, you can create a Point object from a JSON object like thi
Map<String, num> json = {'a': 1, 'b': 2};
Point p = Point.fromJson(json);
You can also use the 'factory' keyword to create a singleton class, which is a class that allows only a single instance to be created.
class Point {
static final Point instance = Point._internal();
num a, b;
factory Point() {
return instance;
}
Point._internal();
}
In this example, calling the Point constructor will always return the same instance of the Point class.
example
import 'dart:math';
class Point {
num a, b;
Point(this.a, this.b);
num distanceTo(Point other) {
var da = a - other.a;
var db = b - other.b;
return sqrt(da * da + db * db);
}
}
void main() {
Point p1 = Point(1, 2);
Point p2 = Point(4, 5);
print(p1.distanceTo(p2)); // Output: 4.47214
}
In the above dart example, the Point class represents a point in two-dimensional space. It has two instance variables, a and b, which represent the coordinates of the point. It also has a method called distanceTo, which calculates the distance between two points.
In the 'main' function, we create two objects of the Point class and then use the distanceTo method to calculate the distance between them.
17. Dart Methods
In the Dart programming language, a method is a function that is defined inside a class or an object. You can use methods to perform operations or calculations on the data stored in an object.
class Point {
num a, b;
Point(this.a, this.b);
num distanceTo(Point other) {
var da = a - other.b;
var da = b - other.b;
return sqrt(da * da + db * db);
}
}
In this example, the Point class has a method called distanceTo that calculates the distance between two points.
You can call a method on an object like this
Point p1 = Point(1, 2);
Point p2 = Point(4, 5);
num distance = p1.distanceTo(p2);
print(distance); // Output: 4.47214
You can also define methods with optional parameters by using the [parameterName] syntax.
class Point {
num a, b;
Point(this.a, this.b);
void move(num da, num db, [bool animate = false]) {
a += da;
b += db;
if (animate) {
// Animate the movement
}
}
}
In this example, the move method has two required parameters, dx and dy, and one optional parameter, animate. You can call this method with or without the optional parameter:
Point p = Point(1, 2);
p.move(3, 4);
p.move(1, 2, true);
18. Dart Inheritance
In the Dart programming language, inheritance is a mechanism that allows a class to inherit properties and methods from a parent class. This allows you to create a new class that is a modified version of an existing class, without having to rewrite all the code in the new class.
class Animal {
String name;
int age;
Animal(this.name, this.age);
void makeSound() {
print('The animal makes a sound');
}
}
class Dog extends Animal {
Dog(String name, int age) : super(name, age);
void makeSound() {
print('The dog barks');
}
void wagTail() {
print('The dog wags its tail');
}
}
class Cat extends Animal {
Cat(String name, int age) : super(name, age);
void makeSound() {
print('The cat meows');
}
void scratch() {
print('The cat scratches');
}
}
void main() {
Animal animal = new Animal('Rex', 5);
animal.makeSound(); // Output: The animal makes a sound
Dog dog = new Dog('Buddy', 3);
dog.makeSound(); // Output: The dog barks
dog.wagTail(); // Output: The dog wags its tail
Cat cat = new Cat('Fluffy', 2);
cat.makeSound(); // Output: The cat meows
cat.scratch(); // Output: The cat scratches
}
In this Dart program, we define a base class Animal with two instance variables name and age, and a method makeSound(). We then define two derived classes Dog and Cat that inherit from the Animal class.
The Dog and Cat classes have their own implementations of the makeSound() method, and also define additional methods wagTail() and scratch(), respectively.
In the main() function, we create instances of the Animal, Dog, and Cat classes and call their methods. Since Dog and Cat inherit from Animal, they also have access to the name and age instance variables and the makeSound() method defined in the Animal class. However, they also have their own unique methods that are not available in the Animal class.
19. Dart enum
In Dart, an enum is a special data type that represents a fixed set of constants. Each constant is assigned an integer value starting from zero, and the values increase by one for each subsequent constant.
Enums are useful for defining a set of related values that are known at compile time, and for ensuring that a value is one of a specified set of values.
enum Color {
red,
green,
blue
}
In this example, we've defined an enum named Color, which contains three constants: red, green, and blue. The integer value of red is 0, green is 1, and blue is 2.
We can also use this enum to create variables and switch statements
Color myColor = Color.green;
switch (myColor) {
case Color.red:
print('The color is red');
break;
case Color.green:
print('The color is green');
break;
case Color.blue:
print('The color is blue');
break;
}
In this example, we've created a variable myColor and assigned it the value Color.green. We then use a switch statement to print out a message depending on the value of myColor. Since myColor is Color.green, the output will be The color is green.
20. Dart Generics
In the Dart programming language, generics are a way to create reusable components that can work with a variety of data types. Generics allow you to specify placeholders for the types of values that a component can accept, so that you can use the same component with different types of values.
class Stack<T> {
List<T> _items = [];
void push(T item) {
_items.add(item);
}
T pop() {
if (_items.isEmpty) {
throw 'Stack is empty';
}
return _items.removeLast();
}
}
In this example, the Stack class is a generic class that can be used with any data type. The placeholder 'T' represents the type of the elements in the stack.
You can use the Stack class like this
Stack<int> stack = Stack<int> ();
stack.push(1);
stack.push(2);
print(stack.pop()); // Output: 2
print(stack.pop()); // Output: 1
ou can also use the shorthand syntax to specify the type of the elements in the stack
Stack<int> stack = Stack();
In this example, the type of the elements in the stack is inferred to be 'int' based on the value passed to the push method.
21. Dart Asynchronus
In the Dart programming language, asynchronous programming allows you to perform tasks concurrently, rather than sequentially. This is useful when you want to perform tasks that take a long time to complete, such as I/O operations or network requests, without blocking the main execution thread.
There are several ways to perform asynchronous tasks in Dart. One way is to use the 'async' and 'await' keywords.
Here is an example of how to use 'async' and 'await' to perform an asynchronous task
import 'dart:io';
Future<void> getData() async {
var url = 'https://example.com/data';
var response = await HttpClient().getUrl(Uri.parse(url));
var contents = await response.transform(utf8.decoder).join();
print(contents);
}
void main() {
getData();
}
In this example, the getData function is an asynchronous function that makes an HTTP request to a URL and prints the response to the console. The 'await' keyword is used to pause the execution of the function until the response is received.
The getData function returns a 'Future' object, which represents a value that may be available in the future. You can use the then method of the 'Future' object to specify a callback function that will be executed when the value is available.
getData().then((value) => print(value));
You can also use the 'async' and 'await' keywords to perform asynchronous tasks in a for loop.
import 'dart:io';
Future<List<int>> getData() async {
var url = 'https://example.com/data';
var response = await HttpClient().getUrl(Uri.parse(url));
return response.transform(utf8.decoder).join();
}
void main() async {
var data = await getData();
for (var i in data) {
print(i);
}
}
In this example, the 'main' function is marked as 'async', which allows it to use the 'await' keyword. The 'for' loop is executed asynchronously, so that each iteration of the loop is performed concurrently.
22. Dart Generators
In the Dart programming language, a generator is a special function that returns an iterable object. You can use a generator to create a sequence of values that can be iterated over, without having to generate the entire sequence in memory at once.
Here is an example of how to define a generator function in Dar
Iterable<int> integers(int start, int end) sync* {
for (int i = start; i <= end; i++) {
yield i;
}
}
In this example, the integers function is a generator function that returns an iterable object containing a range of integers from start to end, inclusive. The 'yield' keyword is used to return a value from the generator function.
You can use the generator function like this
for (int i in integers(1, 10)) {
print(i);
}
This will output the numbers from 1 to 10 to the console.
You can also use the 'sync*' keyword to define a generator function that can be used in a 'sync*' for loop.
Iterable<int> evenIntegers(int start, int end) sync* {
for (int i = start; i <= end; i += 2) {
yield i;
}
}
sync* for (int i in evenIntegers(2, 10)) {
print(i);
}
This will output the even numbers from 2 to 10 to the console.
Thank You
Thank you for visiting 'webdevmonk' to read the Dart tutorial! We hope that you found the tutorial helpful and informative.
If you have any questions or feedback about the tutorial, please don't hesitate to let us know. We are always looking for ways to improve our content and make it more valuable for our readers.
We would also like to invite you to come back and visit webdevmonk again in the future to read more tutorials and learn about other programming languages and technologies. We are constantly adding new content to our website, and we hope you will find it useful in your learning journey.
Thank you for choosing webdevmonk as your learning resource. We hope to see you again soon!